The $ne Operator in MongoDB
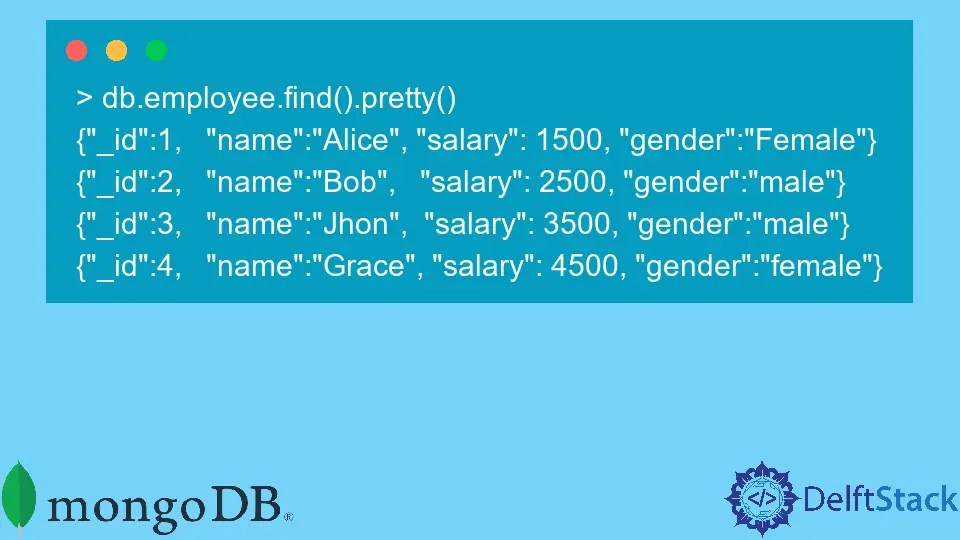
This article will discuss how the $ne
operator works in MongoDB. In addition, we will enumerate its differences with the $not
operator.
the $ne
Operator in MongoDB
$ne
is an operator in MongoDB, which stands for not equal. This compares the value of a field and checks if that is equal.
Syntax:
{field: {$ne: value}}
Suppose you have the following documents in the employee
collection.
> db.employee.find().pretty()
{"_id":1, "name":"Alice", "salary": 1500, "gender":"Female"}
{"_id":2, "name":"Bob", "salary": 2500, "gender":"male"}
{"_id":3, "name":"Jhon", "salary": 3500, "gender":"male"}
{"_id":4, "name":"Grace", "salary": 4500, "gender":"female"}
Now, you want to see all the male
employees. Then, your query should be like following:
> db.employee.find({gender:{$ne: female}}).pretty()
{"_id":2, "name":"Bob", "salary": 2500, "gender":"male"}
{"_id":3, "name":"Jhon", "salary": 3500, "gender":"male"}
Another example, say, you’ve been asked to show the female whose salary is greater than or equal to 4000. So, the query should be:
> db.employee.find({gender:{$ne: male}, salary:{$gte:4000}}).pretty()
{"_id":4, "name":"Grace", "salary": 4500, "gender":"female"}
Difference Between the $not
and the $ne
Operators
You’ve seen the syntax for the $ne
operator above. If you noticed, $ne
only takes values as a parameter.
It simply checks whether a document has the exact value in the given field or not. In some cases, we might need a logical expression rather than a constant value; then, we will use $not
.
Syntax:
{ field: { $not: { <operator-expression> } } }
Here are the similarities and differences between $ne
and $not
:
$ne |
$not |
---|---|
Takes values as a parameter. | Takes logical expression as a parameter. |
Doesn’t support nested query inside it. | You can use nested query inside the $not operator. |
$ne selects the documents where the field’s value is not equal to the specified value, including documents that do not contain the field. |
$not selects the documents that do not match the operator expression, including documents that do not contain the field. |
$ne doesn’t support regex. |
$not supports regex. |
To know more about $ne
and $not
, visit the official documentation attached.