How to Match Multiple Values in MongoDB
- Understanding MongoDB Query Structure
- Using the $in Operator
- Combining Multiple Conditions with $or
- Filtering with $and for Multiple Fields
- Conclusion
- FAQ
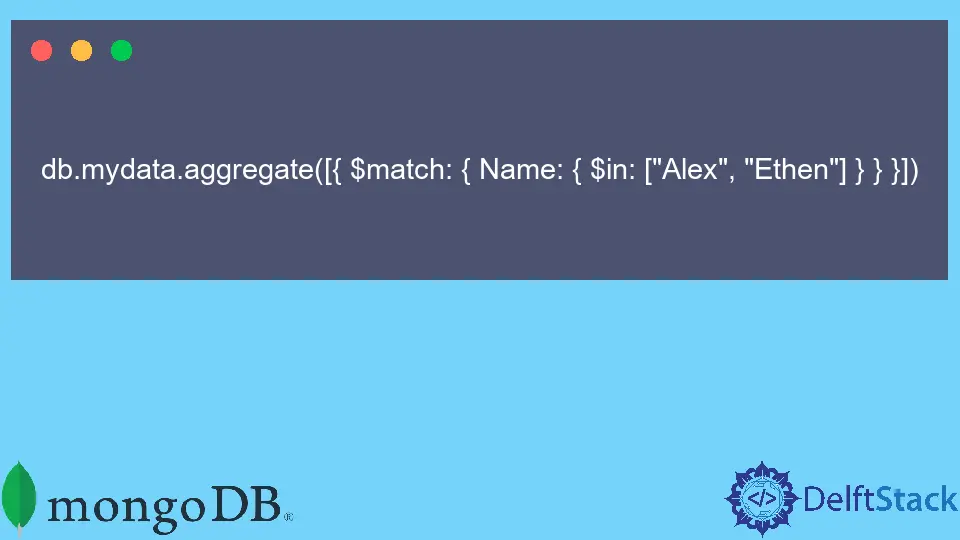
When working with MongoDB, one of the most common tasks is matching multiple values within documents. Whether you’re building a complex application or simply querying your database, knowing how to efficiently retrieve multiple values can save you time and improve performance.
This tutorial will discuss various methods to match multiple values in MongoDB, providing clear examples and explanations to help you understand the process. By the end of this article, you’ll have the tools you need to effectively query your MongoDB collections and retrieve the data you need.
Understanding MongoDB Query Structure
Before diving into the methods for matching multiple values, it’s essential to understand the basic structure of a MongoDB query. MongoDB uses a JSON-like syntax for querying, which makes it intuitive for developers familiar with JavaScript. Queries are typically structured with a collection name followed by a filter object that defines the criteria for matching documents.
For example, a simple query might look like this:
db.collectionName.find({ key: value })
In this structure, collectionName
is the name of your collection, and key: value
defines the criteria for matching documents. Now, let’s explore different methods to match multiple values effectively.
Using the $in Operator
One of the most straightforward ways to match multiple values in MongoDB is by using the $in
operator. This operator allows you to specify an array of values to match against a field in your documents. It’s particularly useful when you want to find documents that contain any of the specified values.
Here’s a simple example using the $in
operator:
db.collectionName.find({ fieldName: { $in: ['value1', 'value2', 'value3'] } })
In this query, fieldName
is the name of the field you’re targeting, and the array contains the values you want to match. This will return all documents where fieldName
is equal to either value1
, value2
, or value3
.
Output:
{
"_id": ObjectId("..."),
"fieldName": "value1",
...
}
{
"_id": ObjectId("..."),
"fieldName": "value2",
...
}
The $in
operator is incredibly powerful and can significantly simplify your queries. It’s especially useful when dealing with large datasets where you need to filter based on multiple criteria. By using this operator, you can ensure that your queries are both concise and efficient.
Combining Multiple Conditions with $or
Another effective method for matching multiple values is using the $or
operator. This operator allows you to combine multiple conditions in a single query, returning documents that meet any of the specified criteria.
Here’s how you can use the $or
operator to match multiple values:
db.collectionName.find({
$or: [
{ fieldName: 'value1' },
{ fieldName: 'value2' },
{ fieldName: 'value3' }
]
})
In this example, the query will return documents where fieldName
is either value1
, value2
, or value3
. The $or
operator is particularly useful when you have complex queries that require multiple conditions to be met.
Output:
{
"_id": ObjectId("..."),
"fieldName": "value1",
...
}
{
"_id": ObjectId("..."),
"fieldName": "value3",
...
}
Using $or
allows for more flexibility in your queries, enabling you to specify various conditions that documents must meet. This can be especially helpful when working with datasets that have diverse attributes and when you need to perform comprehensive searches across multiple fields.
Filtering with $and for Multiple Fields
While the $or
operator is great for matching any of several conditions, the $and
operator is useful when you want to ensure that multiple conditions are met simultaneously. This operator allows you to filter documents based on multiple criteria across different fields.
Here’s an example of how to use the $and
operator:
db.collectionName.find({
$and: [
{ fieldName1: 'value1' },
{ fieldName2: 'value2' }
]
})
In this query, documents will be returned only if they meet both conditions: fieldName1
must equal value1
, and fieldName2
must equal value2
.
Output:
{
"_id": ObjectId("..."),
"fieldName1": "value1",
"fieldName2": "value2",
...
}
The $and
operator is particularly beneficial when you need to narrow down your results based on multiple attributes. By combining conditions, you can create more precise queries that return exactly what you need, making your data retrieval more efficient.
Conclusion
In conclusion, matching multiple values in MongoDB is a fundamental skill that can enhance your data retrieval capabilities. By utilizing operators like $in
, $or
, and $and
, you can create powerful queries that meet your specific needs. Understanding these methods will empower you to work more effectively with your MongoDB collections, ensuring that you can extract the necessary information quickly and efficiently. As you continue to explore MongoDB, remember that the flexibility of its query language allows for a wide range of possibilities in data manipulation and retrieval.
FAQ
-
What is the purpose of the $in operator in MongoDB?
The $in operator is used to match documents where a specified field’s value is in a given array of values. -
Can I combine $or and $and in a single query?
Yes, you can combine both operators in a single query to create complex conditions for filtering documents. -
How does MongoDB handle performance with complex queries?
MongoDB is designed to handle complex queries efficiently, but indexing your fields can significantly enhance performance.
-
What types of data can I match using these operators?
You can match strings, numbers, dates, and other data types supported by MongoDB using these operators. -
Is there a limit to the number of values I can use with the $in operator?
While there isn’t a strict limit, using a very large array may impact performance, so it’s best to keep it reasonable.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn