How to Get Database From Connection String in MongoDB
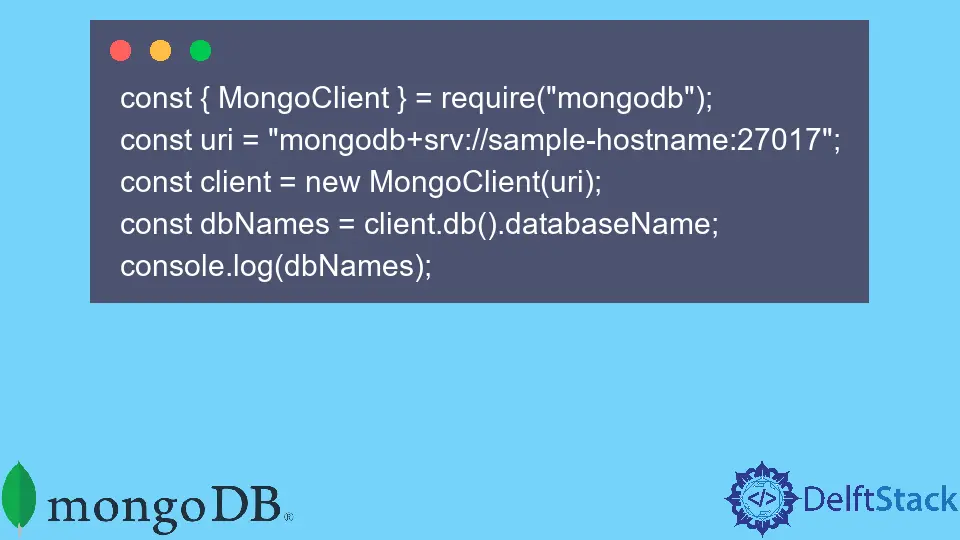
This article will discuss how to get the database name from a connection string in MongoDB.
Get Database From Connection String in MongoDB
The hosts
and settings
to be used are described in the connection string. For this string to be recognized as being in the standard connection format, the prefix mongodb+srv:/
is necessary.
Syntax:
mongodb+srv://[username:password@]host1[:port1][,...[,hostN[:portN]]][/[database][?options]]
The options that the MongoDB connection string offers are as follows.
Options | Description |
---|---|
username |
The username used to connect the driver to Mongo. |
password |
The username used to connect the driver to Mongo. It is an optional parameter. |
host |
It gives the server address to which to connect. |
port |
It gives the port number to use for connecting. 27017 is the default. |
database |
The name of the database driver needs to login to, which is only important when the format username:password@ is used. The "admin" database is used by default if not specified. |
The steps to extract the database name from the MongoDB connection string are listed below.
-
Install
mongodb
package.Install the most recent MongoDB package from
npm
oryarn
in your Node application.npm i `mongodb`
-
Import it into your application.
Use the
require
command to import the most recent version of the MongoDB package. Extract the MongoClient from the package.const {MongoClient} = require('mongodb');
-
Create new mongo client.
By invoking the new
MongoClient()
, a fresh instance of the MongoClient is created, given a URI string.const uri = 'mongodb+srv://sample-hostname:27017'; const client = new MongoClient(uri);
-
Get the database name.
const dbNames = client.db().databaseName;
Full Code Snippet:
const {MongoClient} = require('mongodb');
const uri = 'mongodb+srv://sample-hostname:27017';
const client = new MongoClient(uri);
const dbNames = client.db().databaseName;
console.log(dbNames);
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn