How to Find a Specific Document With Array in MongoDB
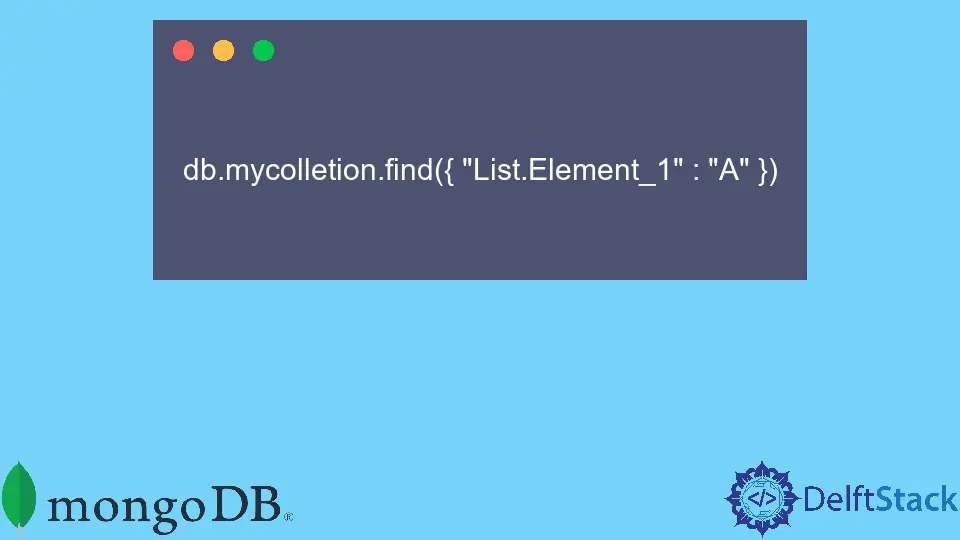
In MongoDB, a field in a document may contain some more data, something like sub-items.
In this article, we will see how we can search a specific document based on the sub-items of a field. Also, we will see an example relevant to the topic to make it easier.
the find()
Method in MongoDB
We use the built-in method find()
to search specific documents from a collection based on specific criteria. Now, we need to pass a parameter that searches specific elements based on the sub-item array.
The general syntax for the parameter will be:
"field.item" : 'value'
Note: Please check if you are in the right collection. To switch on a specific collection, use the command
use YourCollection
.
Find a Specific Document From a Collection in MongoDB
In our below example, we will illustrate how we can find a document from a collection based on the array of items. But first, let’s consider that we have the below documents in our collection:
{
_id: ObjectId("637524953f80c4973e1f16b6"),
sl: 1,
Name: 'Ron',
List: {
Element_1: 'A',
Element_2: '3'
}
}
{
_id: ObjectId("637524953f80c4973e1f16b7"),
sl: 2,
Name: 'Caron',
List: {
Element_1: 'A',
Element_2: '3'
}
}
As you can see above, the field List
contains an array of items as a sub-item. Now, we will find a specific document based on these sub-items.
Now, the command for this purpose will look like this:
db.mycolletion.find({ "List.Element_1" : "A" })
After running the above command, you will get the below output in your console.
{
_id: ObjectId("637524953f80c4973e1f16b6"),
sl: 1,
Name: 'Ron',
List: {
Element_1: 'A',
Element_2: '3'
}
}
Please note that the commands shown in this article are for the MongoDB database and the command needs to be run on the MongoDB console.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn