Compound Index in MongoDB
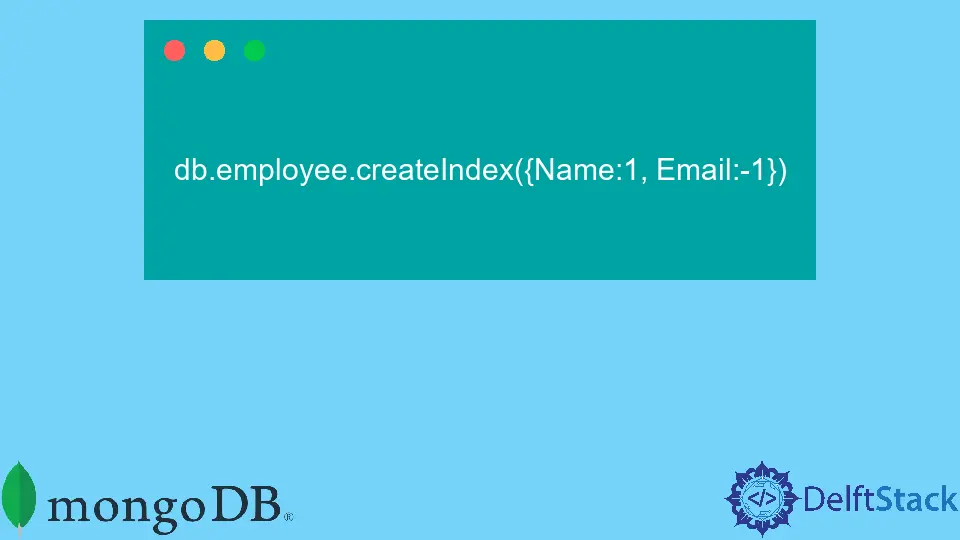
Sometimes we need to create an index that contains multiple fields. For example, if your document contains a field named Sex
, it may contain two other fields, such as Male
and Female
.
These fields may have values like Yes
or No
. In this article, we are going to discuss the compound index, and also we are going to see an example with an explanation to make the topic easier.
MongoDB Index
Indexing is very important as it increases query performance and makes it easier to search for a specific document. A compound index is a kind of index that combines multiple fields.
It is mainly used for these fields that value based on other fields. For example, if you have a field named Name
, then the value of this field may depend on the value of two other fields, FirstName
and LastName
.
So compound index means indexing on multiple fields. The major difference between the General Index (Single Index) and Compound Index is that the Compound Index performs indexing based on multiple values.
Create a Compound Index in MongoDB
MongoDB contains a built-in method named createIndex()
for creating any indexing. To create a compound index, we can follow the general syntax below.
db.collection.createIndex({<field1>: <type1>, <field2>: <type2>, …})
Let’s look at the example below to make it clearer to us.
db.employee.createIndex({Name:1, Email:-1})
In the example above, we created a simple compound index that will sort the employee
collection by the field Name
in ascending order and then the field Email
in descending order.
Before executing any command, please check if you are in the right collection. To switch on a specific collection, use the command
use YourDB
. Otherwise, it will provide you with an error.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn