How to Compare Fields in MongoDB
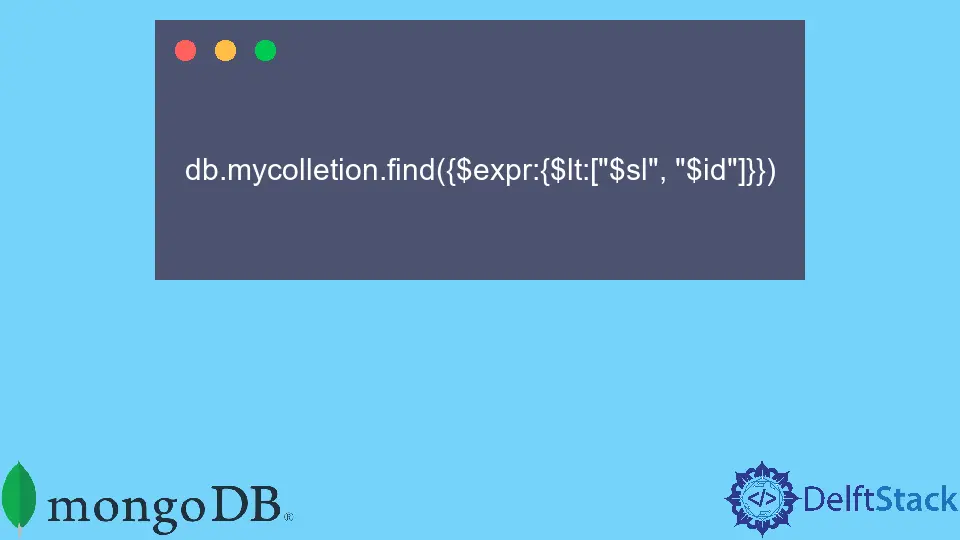
Sometimes, we need to find a document by comparing two fields. For example, we may need to consider the serial and ID numbers when selecting a document in a MongoDB collection; we will see this later in this tutorial.
In this article, we will see how we can compare two fields in MongoDB. Also, we will see a relevant example with an explanation to make the topic easier.
Compare Fields in MongoDB
There are two techniques that we can use for this purpose. We will use the built-in method find()
for both techniques, which is used to search or extract documents from a MongoDB collection.
In our first technique, we will use the keyword $where
that mainly matches specific criteria. And in our second technique, we will use simple aggregation with the keyword $expr
, which allows you to implement aggregation expressions as an argument.
Let’s see an example to make it clearer.
Examples of Comparing Two Fields in MongoDB
In our example below, we will see how we can compare two fields in MongoDB. We will compare the sl
and id
fields.
Now, the commands for this purpose are:
Using the $where
keyword:
db.mycolletion.find( { $where : "this.sl < this.id" } );
Using the $expr
keyword:
db.mycolletion.find({$expr:{$lt:["$sl", "$id"]}})
In the above command, $lt
is a comparison operator. There is some other comparison operator that you can use when necessary.
Available Comparison Operators in MongoDB
Below, we shared the available comparison operators used with aggregation in MongoDB.
$eq
- This represents the Equal operator.$ne
- This represents the Not Equal operator.$gt
- This represents the Greater Than operator.$gte
- This represents the Greater Than or Equal operator.$lt
- This represents the Less Than operator.$lte
- This represents the Less Than or Equal operator.$in
- This matches any values in an array.$nin
- This matches none of the values in an array.
Now, both of the commands shared above will provide you with the same result as the one below:
{ _id: ObjectId("6371fd850f19826ee6ca5138"),
sl: 0,
Name: 'Alen',
id: '3'
}
Note: Please check if you are in the right collection. To switch on a specific collection, use the command
use YourDB
; otherwise, it will give you an error.
Please note that the commands shown in this article are for the MongoDB database and the command needs to be run on the MongoDB console.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn