How to Check if a Document Exists in MongoDB
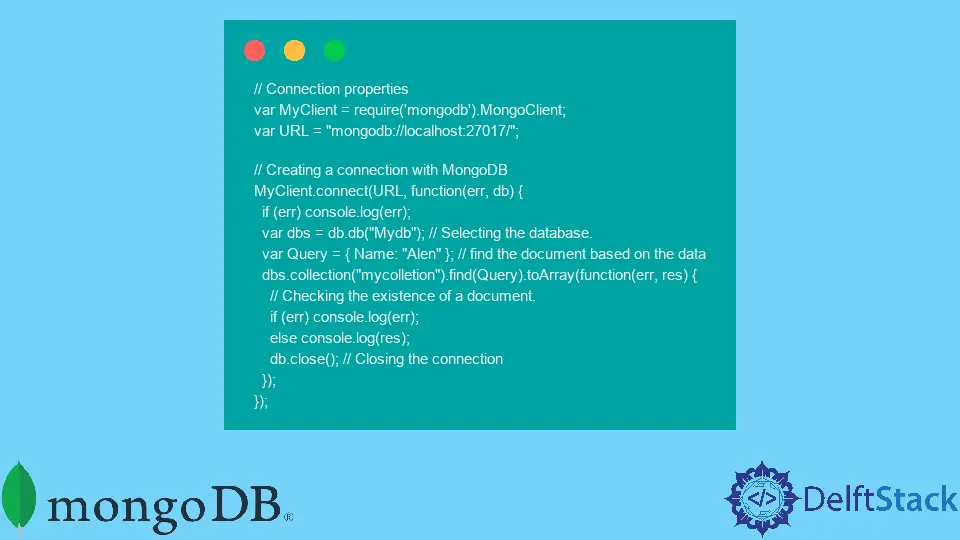
Sometimes, we need to check if the document already exists.
In this article, we will see how we can check if a document already exists in a collection. We will also see a necessary example and explanation to make the topic easy to understand.
Check if a Document Exists in a Collection Using the MongoDB Console
There is a built-in method in MongoDB named find()
for searching specific documents. If the searched document already exists, it will show you the result, but if it doesn’t exist, it won’t show any output.
In this article, we will see two different methods to check the existence of a document.
In our example below, we will use the MongoDB console to find if a document exists. The console command is as follows:
db.mycolletion.find({ Name: "Alen" })
We already discussed the working mechanism of the command. Now, when you run the above command in your console, you will get the below output:
{
_id: ObjectId("6371fd850f19826ee6ca5138"),
sl: 0,
Name: 'Alen',
id: '3'
}
In the next example, we will demonstrate how we can check if a document already exists in a collection from a JavaScript program. The JavaScript code will be as follows:
// Connection properties
var MyClient = require('mongodb').MongoClient;
var URL = 'mongodb://localhost:27017/';
// Creating a connection with MongoDB
MyClient.connect(URL, function(err, db) {
if (err) console.log(err);
var dbs = db.db('Mydb'); // Selecting the database.
var Query = {Name: 'Alen'}; // find the document based on the data
dbs.collection('mycolletion').find(Query).toArray(function(err, res) {
// Checking the existence of a document.
if (err)
console.log(err);
else
console.log(res);
db.close(); // Closing the connection
});
});
In the code above, we already commanded the purpose of each line. Now when you run the above code, you will get the below-resulting output:
[
{
_id: new ObjectId("6371fd850f19826ee6ca5138"),
sl: 0,
Name: 'Alen',
id: '3'
}
]
Please note that we executed the code through Node.js. So you should install the Node.js and the MongoDB package to execute the JavaScript code.
After installing Node.js, you can install MongoDB in your directory using the npm install mongodb
command.
Please note that the commands shown in this article are for the MongoDB database and the command needs to be run on the MongoDB console.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn