How to Check if a Collection Exists in MongoDB Using NodeJS
-
Method 1: Using
listCollections
-
Method 2: Using
collection.countDocuments()
-
Method 3: Using
getCollectionNames
- Conclusion
- FAQ
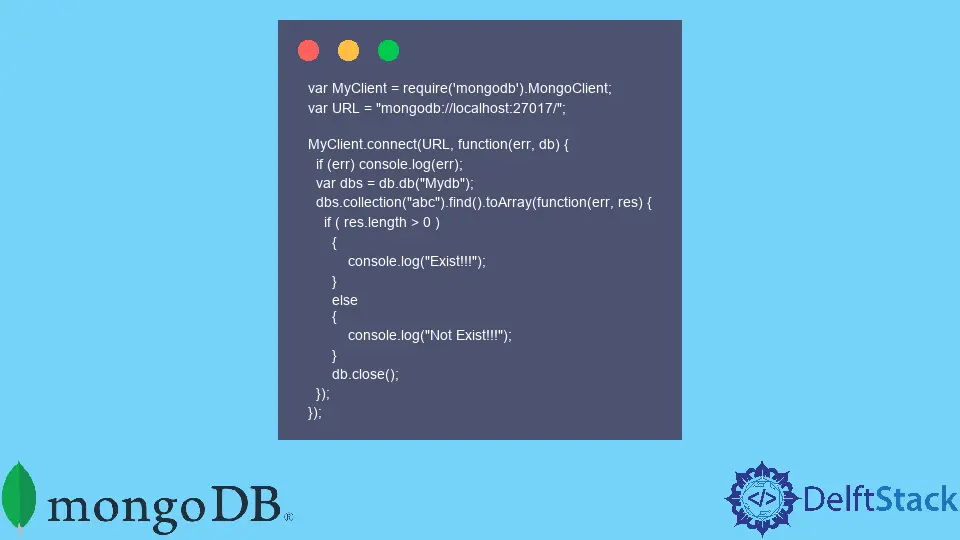
When working with MongoDB in a NodeJS application, it’s essential to manage your collections effectively. One common task developers face is checking whether a specific collection exists before performing operations on it. This can help prevent errors and ensure that your application runs smoothly.
In this tutorial, we will explore how to check if a collection exists in MongoDB using NodeJS. We’ll cover a few straightforward methods and provide code examples to illustrate each approach. By the end of this guide, you’ll have the tools you need to manage your MongoDB collections confidently.
Method 1: Using listCollections
The first method to check if a collection exists in MongoDB is by using the listCollections
command. This command retrieves a cursor containing all collections in the specified database. By iterating through this cursor, you can determine if your desired collection is present.
Here’s a simple code example demonstrating this method:
const { MongoClient } = require('mongodb');
async function checkCollectionExists(dbName, collectionName) {
const uri = 'your_mongodb_connection_string';
const client = new MongoClient(uri);
try {
await client.connect();
const database = client.db(dbName);
const collections = await database.listCollections().toArray();
const collectionExists = collections.some(collection => collection.name === collectionName);
console.log(`Collection "${collectionName}" exists: ${collectionExists}`);
} finally {
await client.close();
}
}
checkCollectionExists('your_database_name', 'your_collection_name');
Output:
Collection "your_collection_name" exists: true/false
In this code, we first establish a connection to the MongoDB server using the MongoClient
. After connecting, we access the specified database and call listCollections()
, which returns an array of the collections. We then use the some
method to check if our desired collection name matches any of the existing collections. Finally, we log the result, indicating whether the collection exists.
Method 2: Using collection.countDocuments()
Another effective way to check for a collection’s existence is by attempting to count the documents in that collection. If the collection does not exist, MongoDB will throw an error, which you can catch to determine the collection’s status.
Here’s how you can implement this method:
const { MongoClient } = require('mongodb');
async function checkCollectionExists(dbName, collectionName) {
const uri = 'your_mongodb_connection_string';
const client = new MongoClient(uri);
try {
await client.connect();
const database = client.db(dbName);
const collection = database.collection(collectionName);
const count = await collection.countDocuments();
console.log(`Collection "${collectionName}" exists with ${count} documents.`);
} catch (error) {
if (error.code === 26) {
console.log(`Collection "${collectionName}" does not exist.`);
} else {
console.error('An error occurred:', error);
}
} finally {
await client.close();
}
}
checkCollectionExists('your_database_name', 'your_collection_name');
Output:
Collection "your_collection_name" exists with X documents.
In this example, we connect to the database and attempt to access the specified collection. We then call countDocuments()
, which returns the number of documents in the collection. If the collection does not exist, MongoDB will throw an error with the code 26
. We catch this error and log a message indicating that the collection does not exist. If the collection exists, we display the count of documents it contains.
Method 3: Using getCollectionNames
The third method involves using the getCollectionNames
function, which retrieves an array of collection names in the specified database. This approach is straightforward and effective for checking the existence of a collection.
Here’s an example of how to use this method:
const { MongoClient } = require('mongodb');
async function checkCollectionExists(dbName, collectionName) {
const uri = 'your_mongodb_connection_string';
const client = new MongoClient(uri);
try {
await client.connect();
const database = client.db(dbName);
const collectionNames = await database.listCollections().toArray();
const exists = collectionNames.some(col => col.name === collectionName);
console.log(`Collection "${collectionName}" exists: ${exists}`);
} finally {
await client.close();
}
}
checkCollectionExists('your_database_name', 'your_collection_name');
Output:
Collection "your_collection_name" exists: true/false
In this code, we use the listCollections()
method to retrieve all collection names in the database. We then check if our target collection name exists in that array. This method is efficient and provides a clear indication of whether the collection is present.
Conclusion
Checking if a collection exists in MongoDB using NodeJS is a crucial skill for any developer working with databases. Whether you choose to use listCollections
, countDocuments
, or getCollectionNames
, each method has its strengths and can be tailored to fit your specific needs. In this tutorial, we explored these methods in detail, providing clear examples to help you implement them in your projects. By incorporating these techniques into your workflow, you can ensure that your applications interact with MongoDB collections smoothly and effectively.
FAQ
- how do I connect to MongoDB using NodeJS?
You can connect to MongoDB using theMongoClient
from themongodb
package. Initialize it with your connection string and call theconnect
method.
-
what should I do if my collection does not exist?
If your collection does not exist, you can create it using thecreateCollection
method or simply start inserting documents, as MongoDB will create the collection automatically. -
can I check for multiple collections at once?
Yes, you can retrieve all collection names and check for multiple collections in a single query by iterating through the array of collection names. -
how can I handle errors when checking for a collection?
Use try-catch blocks to handle any errors that may occur when attempting to access or count documents in the collection. -
what is the best practice for managing collections in MongoDB?
Regularly check for the existence of collections, handle errors gracefully, and ensure that your application logic accounts for potential missing collections.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn