How to Update User Password in MongoDB
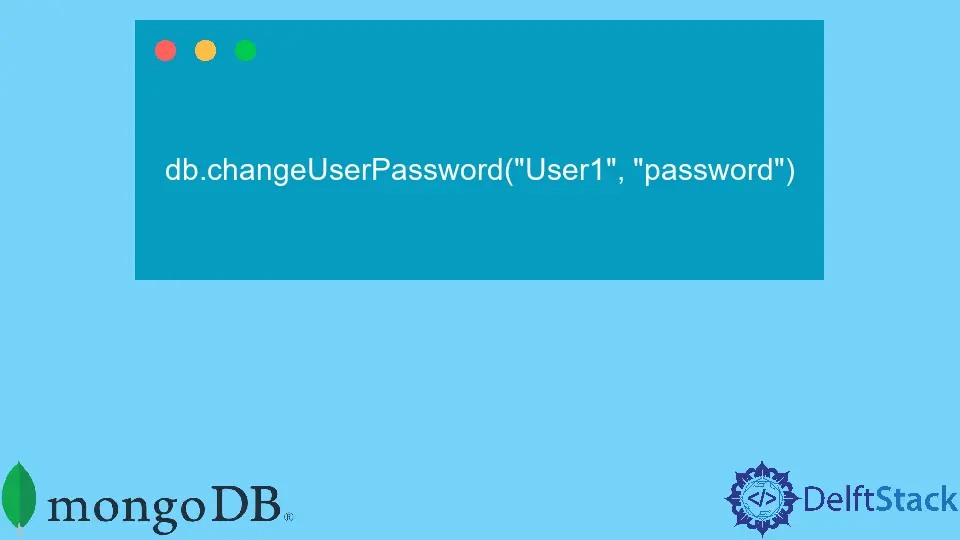
This article will discuss how we can update a user password for the MongoDB database, and also we are going to see an example regarding the topic to make it easier.
Update User Password in MongoDB
Sometimes we need to change the user password mainly for security purposes. There is a built-in command available in MongoDB that allows you to change the user password.
The command follows the below general syntax.
db.changeUserPassword(USERNAME, PASSWORD)
Let’s look at the parameters you need to pass to the command. First, you need to pass the USERNAME
as a string and the PASSWORD
as a string.
To generate a password, you can use the method passwordPrompt()
.
In our below example, we will illustrate how we can change a user’s password in the MongoDB database. The command will be as follows:
db.changeUserPassword("User1", "password")
Now when you run the above command, you will see that the password is updated for the user in your MongoDB database.
Please note that you must provide the username and password as a string in the method
changeUserPassword()
. Otherwise, it will show an error.
Please note that the commands shown in this article are for the MongoDB database and the command needs to be run on the MongoDB console.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn