How to Implement Auto Increment in MongoDB
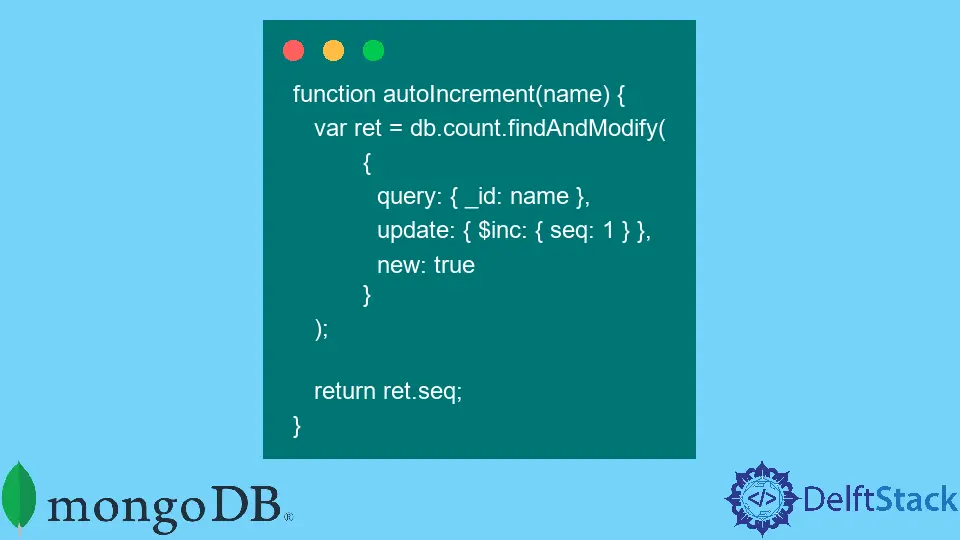
Implementing auto-increment functionality in MongoDB can be a bit tricky since it doesn’t support this feature natively like traditional SQL databases. However, you can achieve it through various methods, ensuring that each document receives a unique, incrementing identifier.
In this tutorial, we will explore how to implement auto-increment in MongoDB effectively. By the end of this article, you will have a solid understanding of the different approaches you can take to manage unique identifiers for your documents. Whether you’re developing a new application or maintaining an existing one, understanding this functionality will enhance your database management skills.
Using a Counter Collection
One of the most common methods to implement auto-increment in MongoDB is by using a separate collection to keep track of the counters. This method involves creating a collection specifically for counters and updating it each time you insert a new document. Here’s how you can do that:
from pymongo import MongoClient
client = MongoClient('mongodb://localhost:27017/')
db = client['mydatabase']
counters = db['counters']
def get_next_sequence(name):
sequence_document = counters.find_one_and_update(
{'_id': name},
{'$inc': {'sequence_value': 1}},
return_document=True,
upsert=True
)
return sequence_document['sequence_value']
# Example of inserting a document with an auto-incremented ID
new_document = {
'_id': get_next_sequence('product_id'),
'name': 'Product A',
'price': 100
}
db['products'].insert_one(new_document)
The code above connects to a MongoDB database and defines a function get_next_sequence
that retrieves and increments the value of a counter. It looks for a document with a specified _id
, increments the sequence_value
, and returns the next value. The upsert=True
option ensures that if the counter does not exist, it will be created. Finally, the example shows how to insert a new product document with an auto-incremented ID.
Output:
{
"_id": 1,
"name": "Product A",
"price": 100
}
This method is efficient and straightforward, allowing you to maintain a unique identifier for each document. However, be cautious about concurrent writes, as this could lead to race conditions. To mitigate this, you may consider using transactions if you are working with MongoDB replica sets.
Using the Aggregation Framework
Another approach to implement auto-increment in MongoDB is by utilizing the aggregation framework. This method is particularly useful when you want to generate a unique identifier based on the existing documents in a collection. Here’s how to do it:
from pymongo import MongoClient
client = MongoClient('mongodb://localhost:27017/')
db = client['mydatabase']
def get_auto_increment_id():
pipeline = [
{'$group': {'_id': None, 'maxId': {'$max': '$_id'}}},
{'$project': {'_id': 0, 'nextId': {'$add': ['$maxId', 1]}}}
]
result = list(db['products'].aggregate(pipeline))
return result[0]['nextId'] if result else 1
# Example of inserting a document with an auto-incremented ID
new_document = {
'_id': get_auto_increment_id(),
'name': 'Product B',
'price': 150
}
db['products'].insert_one(new_document)
In this code snippet, we define a function get_auto_increment_id
that uses an aggregation pipeline to find the maximum _id
in the products
collection. It then adds 1 to this maximum value to generate the next ID. If the collection is empty, it defaults to 1. The example illustrates how to insert a new product document using this auto-incremented ID.
Output:
{
"_id": 2,
"name": "Product B",
"price": 150
}
This method can be advantageous in scenarios where you need to generate IDs based on the current state of the collection. However, keep in mind that this approach may not be suitable for high-concurrency environments, as it does not guarantee uniqueness in real-time.
Conclusion
Implementing auto-increment functionality in MongoDB can be accomplished through various methods, with the most common being the use of a counter collection and the aggregation framework. Each approach has its own advantages and considerations, depending on your application’s specific needs. By understanding these methods, you can effectively manage unique identifiers for your documents, enhancing your database’s integrity and usability. As you dive deeper into MongoDB, keep experimenting with these techniques to find what works best for your projects.
FAQ
-
What is auto-increment in MongoDB?
Auto-increment in MongoDB refers to generating unique, sequential identifiers for documents in a collection. -
Why doesn’t MongoDB support auto-increment natively?
MongoDB is designed for high availability and scalability, which makes implementing traditional auto-increment features challenging. -
Can I use transactions for auto-increment in MongoDB?
Yes, using transactions can help mitigate race conditions when multiple processes attempt to insert documents simultaneously. -
What are the performance implications of using auto-increment?
Auto-increment can introduce performance bottlenecks, especially in high-concurrency environments, so it’s essential to choose the right method. -
Is there a way to reset the auto-increment counter in MongoDB?
Yes, you can manually update the counter document in the counters collection to reset the auto-increment value.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn