How to Save Plot as SVG File in Matplotlib
Maxim Maeder
Feb 02, 2024
Matplotlib
Matplotlib Save
- Generate Data to Create Plots in Matplotlib
-
Use the
savefig
Function to Save Plot as SVG File in Matplotlib
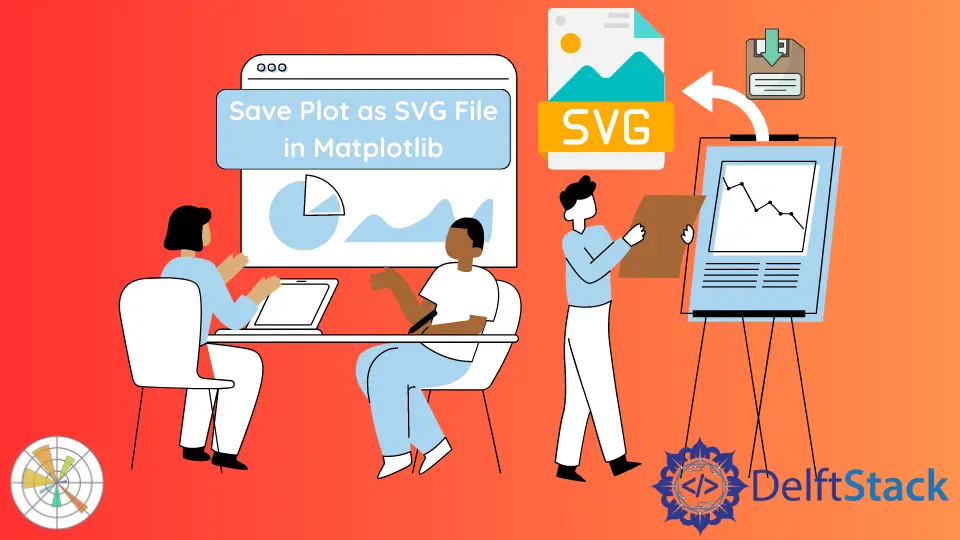
This tutorial teaches you to save figures as Scalable Vector Graphics or SVG.
Generate Data to Create Plots in Matplotlib
Before working with plots, we need to set up our script to work with the library. So we start by importing Matplotlib.
Furthermore, we load the randrange
function from the random
module to quickly generate some data. Keep in mind that it will look different for you.
import matplotlib.pyplot as plt
from random import randrange
data_1 = [randrange(0, 10) for _ in range(0, 10)]
plt.plot(data_1)
Use the savefig
Function to Save Plot as SVG File in Matplotlib
It is easy to save a plot as an SVG file. Just call the savefig
function in the following way.
plt.savefig("plot.svg", format="svg")
After running the code, the figure will be saved to the current directory, which will be scalable.
Complete code:
import matplotlib.pyplot as plt
from random import randrange
data_1 = [randrange(0, 10) for _ in range(0, 10)]
plt.plot(data_1)
plt.savefig("plot.svg", format="svg")
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Author: Maxim Maeder
Hi, my name is Maxim Maeder, I am a young programming enthusiast looking to have fun coding and teaching you some things about programming.
GitHub