Matplotlib Colorbar Range
-
Use the
matpltolib.pyplot.clim()
Function to Set the Range of Colorbar in Matplotlib -
Use the
vmin
andvmax
Parameter to Set the Range of Colorbar in Python
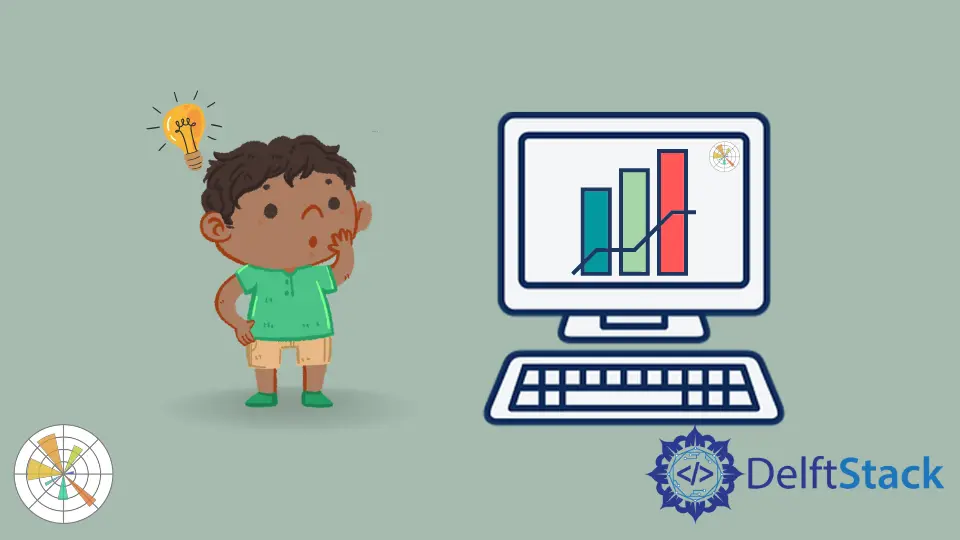
A colorbar can be used to represent the number to a color ratio of the plot. It is like a key showing which numbers are represented in which colors. It is an instance of the plot axes object and can be easily customized.
In this article, we will learn how to set the range of the colorbar in matplotlib figures.
By controlling the range of the colorbar, we can limit color to a particular value range. We will alter the colorbar range of the following graph.
import random
import matplotlib.pyplot as plt
s_x = random.sample(range(0, 100), 20)
s_y = random.sample(range(0, 100), 20)
s = plt.scatter(s_x, s_y, c=s_x, cmap="viridis")
c = plt.colorbar()
Use the matpltolib.pyplot.clim()
Function to Set the Range of Colorbar in Matplotlib
The clim()
function can be used to control the range of the colorbar by setting the color limits of the plot, which are used for scaling.
For example,
import random
import matplotlib.pyplot as plt
s_x = random.sample(range(0, 100), 20)
s_y = random.sample(range(0, 100), 20)
s = plt.scatter(s_x, s_y, c=s_x, cmap="viridis")
c = plt.colorbar()
plt.clim(0, 150)
Notice how the color of the points differs from the original plot by changing the range of the colorbar.
Earlier, the set_clim()
function was also used to achieve this. It was very useful to specify the range of colorbars in multiple subplots. However, this function deprecated in recent versions of the matplotlib library, so it should be avoided.
Use the vmin
and vmax
Parameter to Set the Range of Colorbar in Python
The vmin
and vmax
parameters can be used to specify the scale for mapping color values. These parameters work with the object, which uses colormaps.
It can be used to control the range of the colorbar in matplotlib.
For example,
import random
import matplotlib.pyplot as plt
s_x = random.sample(range(0, 100), 20)
s_y = random.sample(range(0, 100), 20)
s = plt.scatter(s_x, s_y, c=s_x, cmap="viridis", vmin=0, vmax=150)
c = plt.colorbar()
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn