How to Plot Points in Matplotlib
-
Plot Data as Points Using the
matplotlib.pyplot.scatter()
Method -
Plot Data as Points Using the
matplotlib.pyplot.plot()
Method
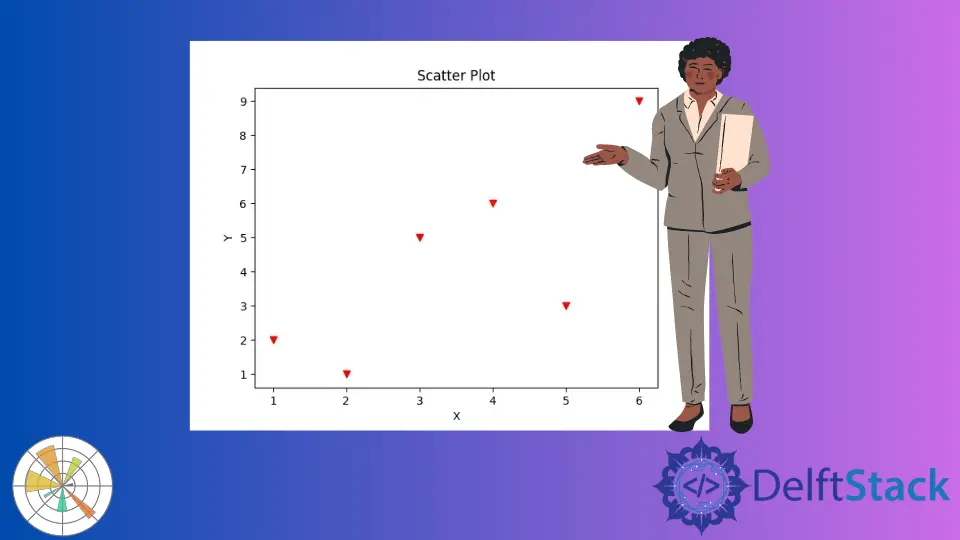
This tutorial explains how we can plot data as points in Matplotlib using the matplotlib.pyplot.scatter()
method and matplotlib.pyplot.plot()
method.
Plot Data as Points Using the matplotlib.pyplot.scatter()
Method
matplotlib.pyplot.scatter()
is the most straightforward and standard method to plot data as points in Matplotlib. We pass the data coordinates to be plotted as arguments to the method.
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5, 6]
y = [2, 1, 5, 6, 3, 9]
plt.scatter(x, y)
plt.xlabel("X")
plt.ylabel("Y")
plt.title("Scatter Plot")
plt.show()
Output:
It generates a simple scatter plot from the given data points. We pass X and Y coordinates as arguments to the scatter()
method to produce the scatter plot. The xlabel()
and the ylabel()
methods will set the X-axis and Y-axis labels respectively. The title()
method will set the title for the figure.
We can also customize the scatter plot by changing color
and marker
parameters to the scatter()
method.
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5, 6]
y = [2, 1, 5, 6, 3, 9]
plt.scatter(x, y, color="red", marker="v")
plt.xlabel("X")
plt.ylabel("Y")
plt.title("Scatter Plot")
plt.show()
Output:
It generates the scatter plot with red color and v
markers.
Plot Data as Points Using the matplotlib.pyplot.plot()
Method
By default, the matplotlib.pyplot.plot()
method will connect all the points with a single line. To generate the scatter plot using the matplotlib.pyplot.plot()
, we set the character to represent the marker as the third argument in the method.
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5, 6]
y = [2, 1, 5, 6, 3, 9]
plt.plot(
x,
y,
"o",
color="red",
)
plt.xlabel("X")
plt.ylabel("Y")
plt.title("Scatter Plot")
plt.show()
Output:
It generates the scatter plot from the data with o
as a marker in red
color to represent data points.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn