Pandas Plot Multiple Columns on Bar Chart With Matplotlib
- Plot Bar Chart of Multiple Columns for Each Observation in the Single Bar Chart
- Stack Bar Chart of Multiple Columns for Each Observation in the Single Bar Chart
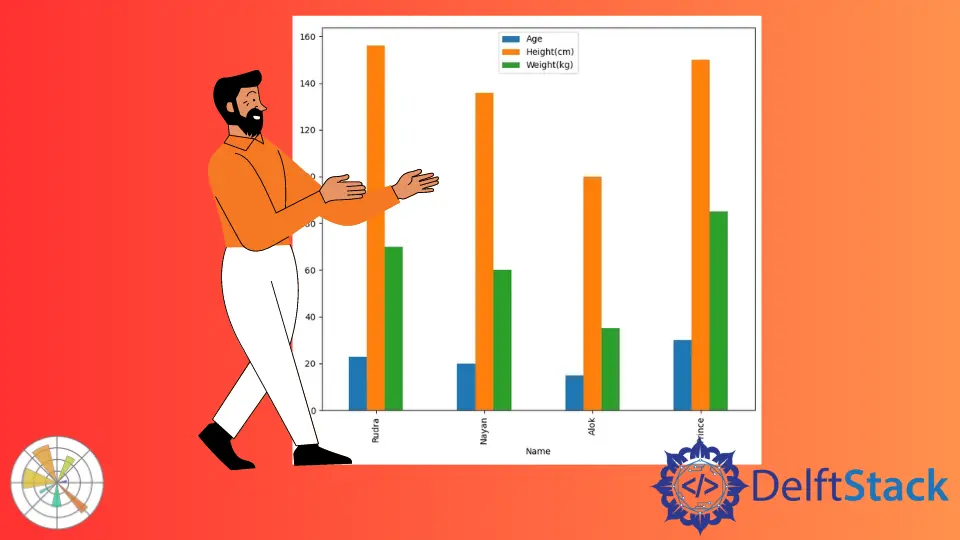
In this tutorial, we will introduce how we can plot multiple columns on a bar chart using the plot()
method of the DataFrame
object.
import pandas as pd
data = [
["Rudra", 23, 156, 70],
["Nayan", 20, 136, 60],
["Alok", 15, 100, 35],
["Prince", 30, 150, 85],
]
df = pd.DataFrame(data, columns=["Name", "Age", "Height(cm)", "Weight(kg)"])
print(df)
Output:
Name Age Height(cm) Weight(kg)
0 Rudra 23 156 70
1 Nayan 20 136 60
2 Alok 15 100 35
3 Prince 30 150 85
We will use the DataFrame df
to construct bar plots. We need to plot age, height, and weight for each person in the DataFrame on a single bar chart.
Plot Bar Chart of Multiple Columns for Each Observation in the Single Bar Chart
import pandas as pd
import matplotlib.pyplot as plt
data = [
["Rudra", 23, 156, 70],
["Nayan", 20, 136, 60],
["Alok", 15, 100, 35],
["Prince", 30, 150, 85],
]
df = pd.DataFrame(data, columns=["Name", "Age", "Height(cm)", "Weight(kg)"])
df.plot(x="Name", y=["Age", "Height(cm)", "Weight(kg)"], kind="bar", figsize=(9, 8))
plt.show()
Output:
It generates a bar chart for Age
, Height
and Weight
for each person in the dataframe df
using the plot()
method for the df
object. We pass a list of all the columns to be plotted in the bar chart as y
parameter in the method, and kind="bar"
will produce a bar chart for the df
. The x
parameter will be varied along the X-axis.
Stack Bar Chart of Multiple Columns for Each Observation in the Single Bar Chart
import pandas as pd
import matplotlib.pyplot as plt
employees = ["Rudra", "Alok", "Prince", "Nayan", "Reman"]
earnings = {
"January": [10, 20, 15, 18, 14],
"February": [20, 13, 10, 18, 15],
"March": [20, 20, 10, 15, 18],
}
df = pd.DataFrame(earnings, index=employees)
df.plot(kind="bar", stacked=True, figsize=(10, 8))
plt.legend(loc="lower left", bbox_to_anchor=(0.8, 1.0))
plt.show()
Output:
It displays the bar chart by stacking one column’s value over the other for each index in the DataFrame.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn