How to Create a Normalized Histogram Using Python Matplotlib
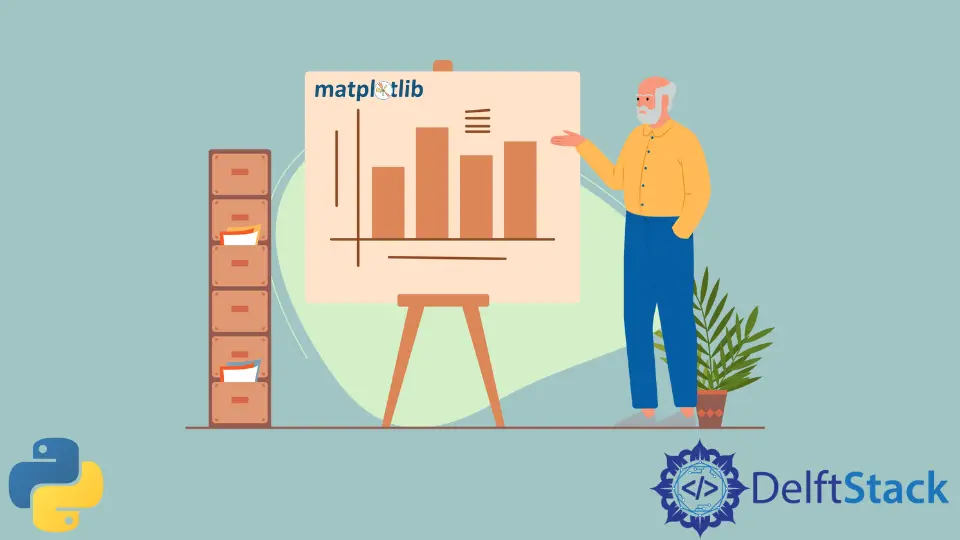
A histogram is a frequency distribution that depicts the frequencies of different elements in a dataset. This graph is generally used to study frequencies and determine how the values are distributed in a dataset.
Normalization of histogram refers to mapping the frequencies of a dataset between the range [0, 1]
both inclusive. In this article, we will learn how to create a normalized histogram in Python.
Create a Normalized Histogram Using the Matplotlib
Library in Python
The Matplotlib
module is a comprehensive Python module for creating static and interactive plots. It is a very robust and straightforward package that is widely used in data science for visualization purposes. Matplotlib
can be used to create a normalized histogram. This module has a hist()
function. that is used for creating histograms. Following is the function definition of the hist()
method.
matplotlib.pyplot.hist(x, bins=None, range=None, density=False, weights=None, cumulative=False, bottom=None, histtype='bar', align='mid', orientation='vertical', rwidth=None, log=False, color=None, label=None, stacked=False, *, data=None, **kwargs)
Following is a brief explanation of the arguments we will use to generate a normalized histogram.
x
: A list, a tuple, or a NumPy array of input values.density
: A boolean flag for plotting normalized values. By default, it isFalse
.color
: The colour of the bars in the histogram.label
: A label for the plotted values.
Refer to the following Python code to create a normalized histogram.
import matplotlib.pyplot as plt
x = [1, 9, 5, 7, 1, 1, 2, 4, 9, 9, 9, 3, 4, 5, 5, 5, 6, 5, 5, 7]
plt.hist(x, density=True, color="green", label="Numbers")
plt.legend()
plt.show()
Output: