Named Colors in Matplotlib
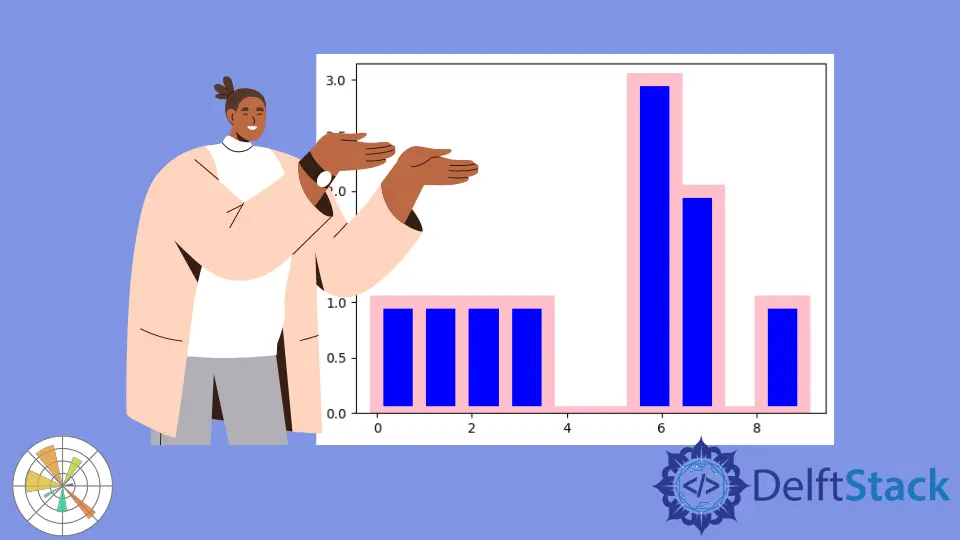
This tutorial teaches you what the named colors in Matplotlib are and how to use them.
Before coloring our plots, we need to set up our script to work with the library. Copy and paste the code below, and keep in mind that your outputs will differ.
import matplotlib.pyplot as plt
from random import randrange
data_1 = [randrange(0, 10) for _ in range(0, 10)]
data_2 = [randrange(0, 10) for _ in range(0, 10)]
Overview of the CSS Colors
There are many ways to define the Plot color in Matplotlib, and now we will look at named colors. The following Picture shows all CSS named Colors available for usage.
Use the Colors in Plots
Line Color
Defining colors is as easy as passing the color keyword argument a color name as a string. I used red and blue for two randomly generated lines in the following example.
plt.plot(data_1, color="red")
plt.plot(data_2, color="blue")
plt.show()
Output:
Edge Color
There is also the option to change the outline of certain graph types by filling the ec
keyword argument. We also set the line width in the following example to see the difference.
plt.hist(data_2, color="blue", ec="pink", lw=10)
Output:
Marker Color
Using markers makes it possible to change their face and edge color with the mfc
/markerfacecolor
and the mec
/markeredgecolor
keyword arguments.
plt.plot(data_1, color="steelblue", marker="o", mec="tomato", markerfacecolor="tomato")
Output:
Complete code:
import matplotlib.pyplot as plt
from random import randrange
data_1 = [randrange(0, 10) for _ in range(0, 10)]
data_2 = [randrange(0, 10) for _ in range(0, 10)]
plt.plot(data_1, color="steelblue", marker="o", mec="tomato", markerfacecolor="tomato")
plt.hist(data_2, color="blue", ec="pink", lw=10)
plt.show()
Hi, my name is Maxim Maeder, I am a young programming enthusiast looking to have fun coding and teaching you some things about programming.
GitHub