Latex Formulas in Matplotlib
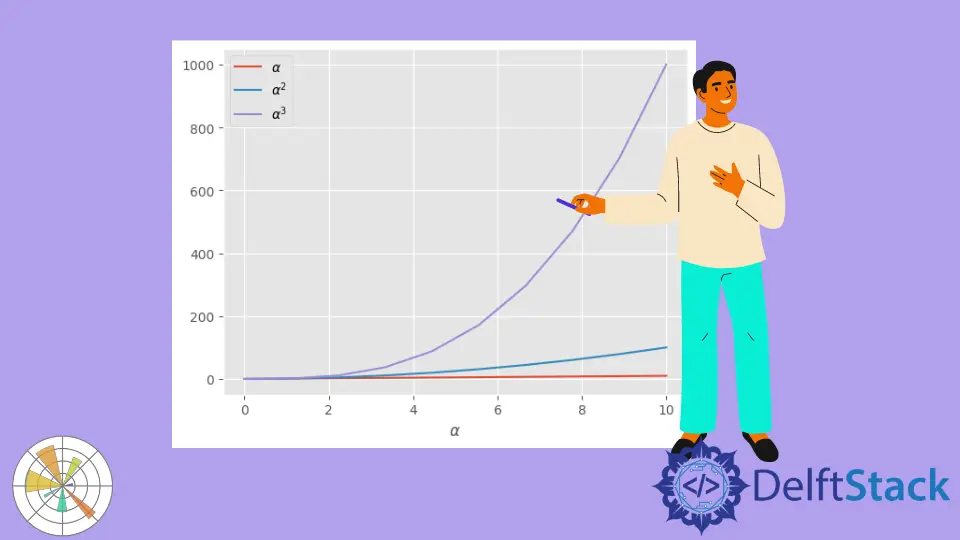
This tutorial explains how we can render the LaTex
formulas or equations in Matplotlib.
Write LaTex
Formulae in Matplotlib Python
import math
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(0, 2 * math.pi, 100)
y = np.sin(x)
plt.plot(x, y)
plt.xlabel("x")
plt.ylabel(r"$\sin (x)$")
plt.title("Plot of sinx")
plt.show()
Output:
It will render the LaTex
formulae in the Matplotlib figure.
To render a LaTex
formula in Matplotlib, we must set 'text.usetex'
to True
. We can use the following script to check the value of 'text.usetex'
:
import matplotlib
print(matplotlib.rcParams["text.usetex"])
Output:
False
You may get True
as the output if 'text.usetex'
to True
for your system. If 'text.usetex'
is set to False
, we can use the following script to set 'text.usetex'
to True
:
import matplotlib
matplotlib.rcParams["text.usetex"] = True
We also need to have LaTex
, dvipng
and Ghostscript
(Version 9.0 or later) to render the LaTex
formulae and add all the installations dependencies to the PATH
.
We can also render Greek alphabets and many more symbols in Matplotlib using the Tex
format.
import numpy as np
import matplotlib.pyplot as plt
alpha = x = np.linspace(0, 10, 10)
y1 = alpha
y2 = alpha ** 2
y3 = alpha ** 3
plt.plot(x, y1, label=r"$\alpha$")
plt.plot(x, y2, label=r"$\alpha^2$")
plt.plot(x, y3, label=r"$\alpha^3$")
plt.xlabel(r"$\alpha$")
plt.legend()
plt.show()
Output:
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn