How to Label Lines in Matplotlib
-
Method 1: Using the
label
Parameter - Method 2: Adding Text Annotations
- Method 3: Customizing Legend Location and Appearance
-
Method 4: Using
ax.annotate
for Dynamic Labels - Conclusion
- FAQ
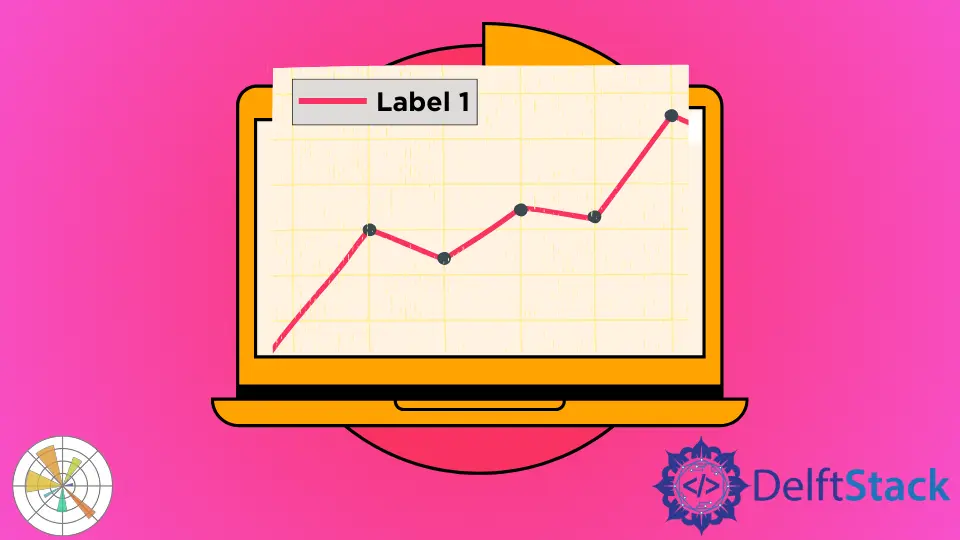
Matplotlib is a powerful library in Python that allows for comprehensive data visualization. One of the essential features that can enhance your plots is the ability to label lines effectively. Labeling lines not only makes your graphs more informative but also helps viewers understand the data presented.
In this article, we will explore various methods to label lines in Matplotlib, providing clear examples and explanations to ensure you can implement these techniques in your projects. Whether you’re creating simple line charts or complex visualizations, mastering line labeling will significantly improve the clarity and professionalism of your work. Let’s dive into the different ways to label lines in Matplotlib!
Method 1: Using the label
Parameter
One of the simplest ways to label lines in Matplotlib is by using the label
parameter in the plotting function. This method is straightforward and effective for basic line plots. When you specify a label for a line, you can then display it in a legend, making your plot easier to read.
Here’s how you can do it:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y1 = [2, 3, 5, 7, 11]
y2 = [1, 4, 6, 8, 10]
plt.plot(x, y1, label='Prime Numbers', color='blue')
plt.plot(x, y2, label='Even Numbers', color='orange')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Line Plot Example')
plt.legend()
plt.show()
Output:
A line plot with two labeled lines: Prime Numbers and Even Numbers.
In this example, we have two lines representing different sets of numbers. By passing the label
parameter to each plt.plot()
call, we can identify each line in the resulting legend. The plt.legend()
function is then called to display the legend on the plot. This method is particularly useful when comparing multiple datasets, as it provides immediate context for each line.
Method 2: Adding Text Annotations
Another effective way to label lines is by using text annotations directly on the plot. This method allows you to place labels at specific coordinates, giving you more control over the positioning and appearance of your labels. This can be particularly useful if you want to highlight specific points or trends in your data.
Here’s an example:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
plt.plot(x, y, color='green')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Line Plot with Annotations')
for i, value in enumerate(y):
plt.text(x[i], value, f'({x[i]}, {value})', fontsize=9, ha='right')
plt.show()
In this example, we use the plt.text()
function to place annotations next to each data point on the line. The ha
parameter controls the horizontal alignment of the text, while the fontsize
parameter adjusts the size of the text. This method is particularly useful for emphasizing specific data points or trends without relying solely on a legend.
Method 3: Customizing Legend Location and Appearance
While the basic legend functionality is great, sometimes you need more customization. Matplotlib allows you to modify the appearance and location of your legends, ensuring they fit well within your plots and do not obstruct important data.
Here’s how you can customize your legend:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y1 = [2, 3, 5, 7, 11]
y2 = [1, 4, 6, 8, 10]
plt.plot(x, y1, label='Prime Numbers', color='blue', linestyle='--', linewidth=2)
plt.plot(x, y2, label='Even Numbers', color='orange', linestyle=':', linewidth=2)
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Customized Legend Example')
plt.legend(loc='upper left', frameon=True, shadow=True, fontsize='small')
plt.show()
Output:
A line plot with a customized legend located in the upper left corner.
In this example, we customized the legend by changing its location to the upper left corner. We also added a frame and shadow to the legend for better visibility. The fontsize
parameter allows us to adjust the text size, making it more readable. Customizing legends is particularly useful when you have multiple lines and want to ensure clarity without cluttering the plot.
Method 4: Using ax.annotate
for Dynamic Labels
For more advanced labeling, you can use the ax.annotate
method, which provides even greater flexibility. This method allows you to create dynamic labels that can point to specific data points or regions on your plot.
Here’s an example of how to use ax.annotate
:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
fig, ax = plt.subplots()
ax.plot(x, y, color='purple')
ax.set_xlabel('X-axis')
ax.set_ylabel('Y-axis')
ax.set_title('Dynamic Annotation Example')
ax.annotate('Peak Value', xy=(4, 7), xytext=(3, 8),
arrowprops=dict(facecolor='black', shrink=0.05))
plt.show()
Output:
A line plot with a dynamic annotation pointing to the peak value.
In this example, the ax.annotate()
function is used to create a label that points to the peak value of the line. The xy
parameter specifies the point being labeled, while xytext
indicates where the text will appear. The arrowprops
dictionary allows you to customize the appearance of the arrow pointing to the label. This method is particularly effective for highlighting significant features in your data, making your visualizations more informative.
Conclusion
Labeling lines in Matplotlib is a crucial skill for anyone looking to create effective data visualizations. By utilizing the various methods outlined in this article—from simple labels to dynamic annotations—you can enhance the clarity and professionalism of your plots. Whether you are creating reports, presentations, or exploratory data analyses, mastering these techniques will significantly improve your audience’s understanding of the data. With practice, you’ll find that labeling becomes an intuitive part of your plotting process, allowing your visualizations to speak volumes.
FAQ
-
How do I change the color of the labels in Matplotlib?
You can change the color of the labels by using thecolor
parameter within theplt.text()
orax.annotate()
functions. -
Can I add multiple legends to a single plot?
While Matplotlib supports only one legend per axes by default, you can create multiple legends by using additional axes or customizing your legend entries. -
What is the difference between
plt.legend()
andax.legend()
?
plt.legend()
is a function from the pyplot interface, whileax.legend()
is a method of the Axes class. Both serve the same purpose but are used in different contexts. -
Is it possible to format the text in annotations?
Yes, you can format the text in annotations using HTML-like syntax for fonts or by using Matplotlib’s text properties likefontsize
,fontweight
, andfontstyle
. -
How do I remove the legend from my plot?
You can remove the legend by not callingplt.legend()
orax.legend()
, or you can set the legend toNone
.
Hi, my name is Maxim Maeder, I am a young programming enthusiast looking to have fun coding and teaching you some things about programming.
GitHub