How to Plot Two Histograms Together in Matplotlib
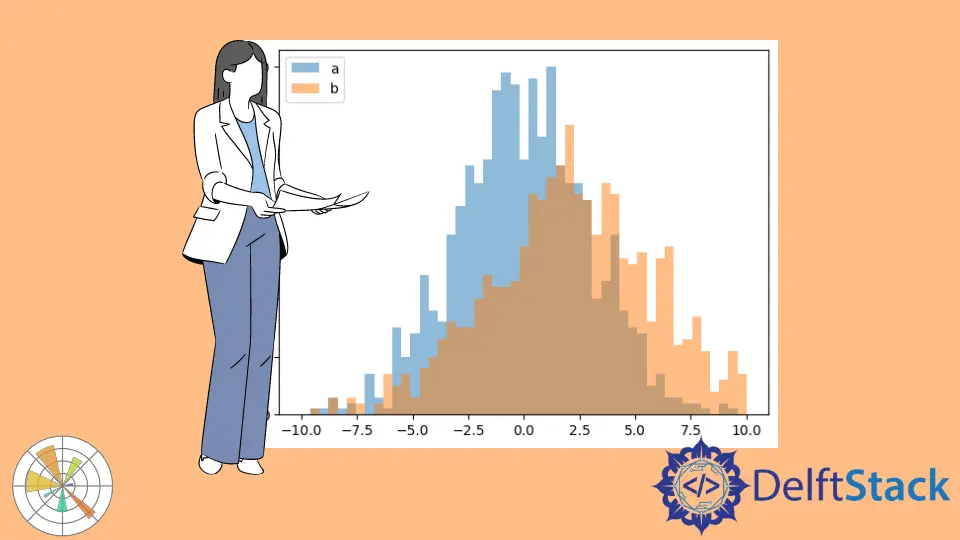
We could plot two histograms in one figure at the same time. Below shows methods to create the two histograms with and without overlapping bars.
Two Histograms Without Overlapping Bars
Working Example Codes:
import numpy as np
import matplotlib.pyplot as plt
a = np.random.normal(0, 3, 3000)
b = np.random.normal(2, 4, 2000)
bins = np.linspace(-10, 10, 20)
plt.hist([a, b], bins, label=["a", "b"])
plt.legend(loc="upper left")
plt.show()
Two Histograms With Overlapping Bars
Working Example Codes:
import numpy as np
import matplotlib.pyplot as plt
a = np.random.normal(0, 3, 1000)
b = np.random.normal(2, 4, 900)
bins = np.linspace(-10, 10, 50)
plt.hist(a, bins, alpha=0.5, label="a")
plt.hist(b, bins, alpha=0.5, label="b")
plt.legend(loc="upper left")
plt.show()
When we call plt.hist
twice to plot the histograms individually, the two histograms will have the overlapped bars as you could see above.
The alpha
property specifies the transparency of the plot. 0.0
is transparent and 1.0
is opaque.
When alpha
is set to be 0.5 for both histograms, the overlapped area shows the combined color. But if alpha
is 0.0
, the default value, overlapped bar only shows the color of the higher value among two histograms and the other color is hidden, as shown below.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook