How to Change the Figure Size in Matplotlib
- Method 1: Using the figsize Parameter in plt.figure()
- Method 2: Adjusting the Figure Size After Creation
- Method 3: Using the rcParams to Set Default Figure Size
- Conclusion
- FAQ
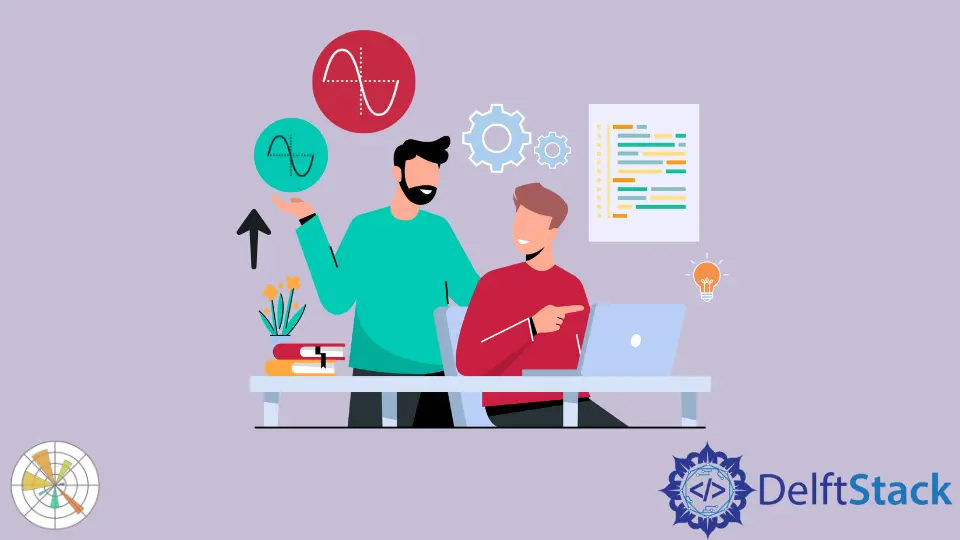
Matplotlib is a powerful plotting library in Python that allows users to create a wide variety of static, animated, and interactive visualizations. One common task that often arises when working with Matplotlib is adjusting the figure size to ensure that your plots are visually appealing and fit well within your presentation or report.
In this tutorial, we will explore how to change the figure size in Matplotlib using simple methods. Whether you’re looking to make a small adjustment or create a large, detailed figure, this guide will walk you through the steps. Let’s dive in and discover how easy it is to customize your Matplotlib figures!
Method 1: Using the figsize Parameter in plt.figure()
One of the simplest ways to change the figure size in Matplotlib is by using the figsize
parameter in the plt.figure()
function. This method allows you to specify the width and height of the figure in inches.
Here’s how you can do it:
import matplotlib.pyplot as plt
plt.figure(figsize=(10, 5))
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.title("Sample Plot with Custom Figure Size")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.grid()
plt.show()
In this example, we create a figure with a width of 10 inches and a height of 5 inches. The figsize
parameter takes a tuple, where the first value represents the width and the second value represents the height. This method is particularly useful when you want to ensure that your plots are not cramped or overly stretched, allowing for better readability and presentation.
Method 2: Adjusting the Figure Size After Creation
Sometimes, you may want to change the size of your figure after it has already been created. Matplotlib provides a straightforward way to do this using the set_size_inches()
method. This method allows you to adjust the figure size dynamically, which can be useful in interactive environments or when working with multiple plots.
Here’s how to use it:
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 9, 16])
fig.set_size_inches(12, 6)
plt.title("Adjusted Plot Size")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.grid()
plt.show()
In this example, we first create a figure and an axis using plt.subplots()
. After plotting our data, we call fig.set_size_inches(12, 6)
, which changes the figure size to 12 inches wide and 6 inches tall. This method is beneficial when you need to make adjustments on the fly, ensuring that your visualizations always look their best.
Method 3: Using the rcParams to Set Default Figure Size
If you frequently find yourself needing to set the same figure size for multiple plots, you can use Matplotlib’s rcParams
to set a default figure size. This way, every plot you create will automatically use the specified size unless overridden.
Here’s an example of how to set this up:
import matplotlib.pyplot as plt
plt.rcParams['figure.figsize'] = [8, 4]
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.title("Default Figure Size")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.grid()
plt.show()
In this example, we set the default figure size to 8 inches wide and 4 inches tall by modifying the plt.rcParams
dictionary. This approach is highly efficient, especially for projects involving numerous plots, as it saves you from having to specify the figure size each time. You can always revert to the default settings or adjust them as needed, giving you flexibility in your visualizations.
Conclusion
Changing the figure size in Matplotlib is a straightforward process that can significantly enhance the quality and clarity of your visualizations. Whether you choose to set the size when creating the figure, adjust it afterwards, or establish a default size for all your plots, each method offers unique advantages. By mastering these techniques, you’ll be well-equipped to create professional-looking plots that effectively communicate your data. Happy plotting!
FAQ
-
How do I set a specific size for a single plot in Matplotlib?
You can set a specific size for a single plot by using thefigsize
parameter in theplt.figure()
function. -
Can I change the figure size after creating a plot?
Yes, you can change the figure size after creating a plot using theset_size_inches()
method on the figure object. -
How can I set a default figure size for all my plots?
You can set a default figure size for all your plots by modifying theplt.rcParams['figure.figsize']
parameter. -
What units are used for the figure size in Matplotlib?
The figure size in Matplotlib is specified in inches.
- Why is it important to adjust figure size in Matplotlib?
Adjusting the figure size is important for improving readability and presentation, ensuring that your visualizations effectively communicate the intended message.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook