How to Add a Y-Axis Label to the Secondary Y-Axis in Matplotlib
Suraj Joshi
Feb 02, 2024
-
Direct Interaction With
axes
Object -
DataFrame.plot
Method to Add a Y-Axis Label to the Secondary Y-Axis
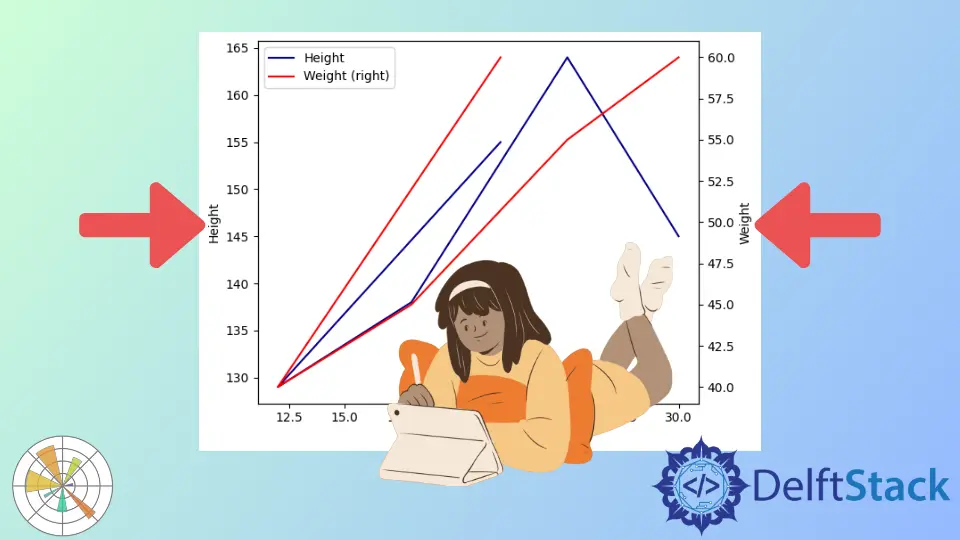
We can interact with the axes
object directly or use DataFrame.plot
method to add a y-axis label to the secondary y-axis in Matplotlib.
Direct Interaction With axes
Object
We can make a plot with two different y-axis by using two different axes
objects with the help of twinx()
function. Now we can use the second axes
object to make the plot of the second y-axis variable and update its label.
import numpy as np
import matplotlib.pyplot as plt
a = np.linspace(0, 5, 100)
y1 = np.sin(2 * np.pi * a)
y2 = np.cos(2 * np.pi * a)
fig, ax1 = plt.subplots()
ax1.set_xlabel("time (s)")
ax1.set_ylabel("sin", color="red")
ax1.plot(a, y1, color=color)
ax1.tick_params(axis="y", labelcolor=color)
ax2 = ax1.twinx()
ax2.set_ylabel("cos", color="green")
ax2.plot(a, y2, color=color)
ax2.tick_params(axis="y", labelcolor=color)
fig.tight_layout()
plt.show()
Output:
ax2 = ax1.twinx()
Axes.twinx()
creates a new Axes
with a y-axis that is opposite to the original axis, in this example ax1
.
DataFrame.plot
Method to Add a Y-Axis Label to the Secondary Y-Axis
We can add a y-axis label to the secondary y-axis with pandas
too. When we set the secondary_y
option to be True
in DataFrame.plot
method, it returns different axes that can be used to set the labels.
import pandas as pd
import matplotlib.pyplot as plt
df = pd.DataFrame(
{
"Age": [22, 12, 18, 25, 30],
"Height": [155, 129, 138, 164, 145],
"Weight": [60, 40, 45, 55, 60],
}
)
ax = df.plot(kind="line", x="Age", y="Height", color="DarkBlue")
ax2 = df.plot(kind="line", x="Age", y="Weight", secondary_y=True, color="Red", ax=ax)
ax.set_ylabel("Height")
ax2.set_ylabel("Weight")
plt.tight_layout()
plt.show()
Output:
Author: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn