How to Display an Image With Matplotlib Python
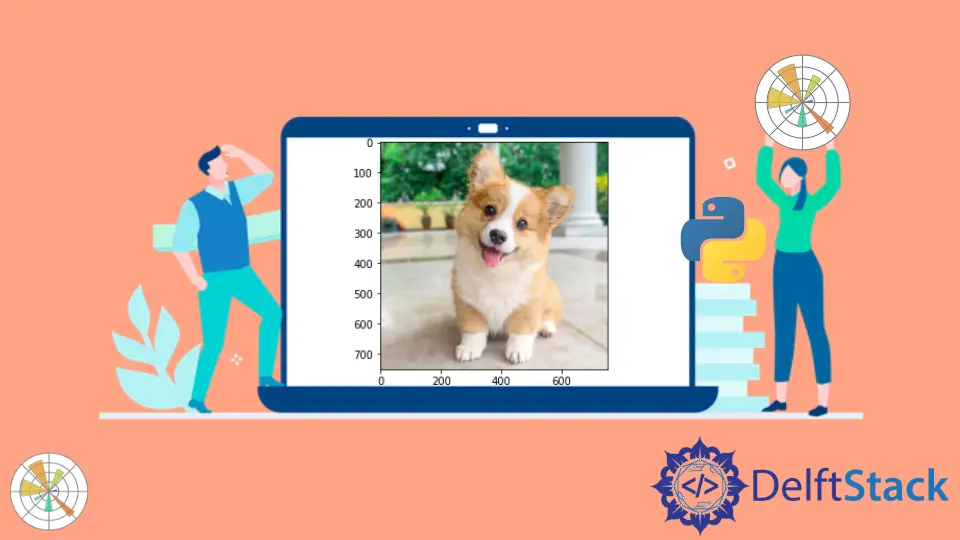
This tutorial discusses how you can use the matplotlib.pyplot.imshow()
method to display images using Matplotlib.
matplotlib.pyplot.imshow()
Method
matplotlib.pyplot.imshow()
displays figures in Matplotlib.
matplotlib.pyplot.imshow()
Syntax
matplotlib.pyplot.imshow(X,
cmap=None,
norm=None,
aspect=None,
interpolation=None,
alpha=None,
vmin=None,
vmax=None,
origin=None,
extent=None, *,
filternorm=True,
filterrad=4.0,
resample=None,
url=None,
data=None,
**kwargs)
Here, X
represents an array-like structure of the image displayed or the PIL
Image.
Examples: Display an Image With Matplotlib Python Using imshow()
import matplotlib.pyplot as plt
import matplotlib.image as img
image = img.imread("lena.jpg")
plt.imshow(image)
plt.show()
Output:
It reads the image lena.jpg
from the current working directory using the imread()
method from the matplotlib.image
module and then finally displays the image using the imshow()
method. You must call the show()
method after imshow()
to view the picture if you’re not using IPython Notebooks
; the show()
method will launch a separate window of the image.
Examples: Display a PIL
Image With Matplotlib Python Using imshow()
import matplotlib.pyplot as plt
from PIL import Image
image = Image.open("lena.jpg")
plt.imshow(image)
plt.show()
Output:
It displays the PIL
image. We read it using the open()
method from the Image
module of PIL
. We can also directly display the image using PIL
in a much simpler way.
from PIL import Image
img = Image.open("lena.jpg")
img.show()
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn