How to Plot Quiver Diagram in Matplotlib
Maxim Maeder
Feb 15, 2024
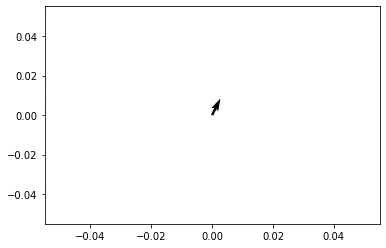
This Tutorial teaches you how to make quiver diagrams with Matplotlib.
Create Quiver Diagram With Matplotlib
We need to supply the matplotlib.pyplot.quiver
function with four arguments to make the diagram. The X, Y-axis, and the X, Y vector direction.
The x coordinate is the first item of the coordinates array, and the second item is the Y coordinate, respectively. It’s the same for the next array, which represents the vector.
Code:
import matplotlib.pyplot as plt
coordinates = [0, 0]
vector = [1, 2]
plt.quiver(coordinates[0], coordinates[1], vector[0], vector[1])
Output:
We can also make multiple arrows that point in different directions. Remember that the arrays all need to have the same amount of items or length.
Code:
import matplotlib.pyplot as plt
from random import randrange
x = [x for x in range(10)]
y = [y for y in range(10)]
vec_x = [randrange(-2, 2) for x in range(10)]
vec_y = [randrange(-2, 2) for x in range(10)]
plt.quiver(x, y, vec_x, vec_y)
Output:
Complete Code:
import matplotlib.pyplot as plt
from random import randrange
x = [x for x in range(10)]
y = [y for y in range(10)]
vec_x = [randrange(-2, 2) for x in range(10)]
vec_y = [randrange(-2, 2) for x in range(10)]
plt.quiver(x, y, vec_x, vec_y)
Author: Maxim Maeder
Hi, my name is Maxim Maeder, I am a young programming enthusiast looking to have fun coding and teaching you some things about programming.
GitHub