How to Add Trendline in Python Matplotlib
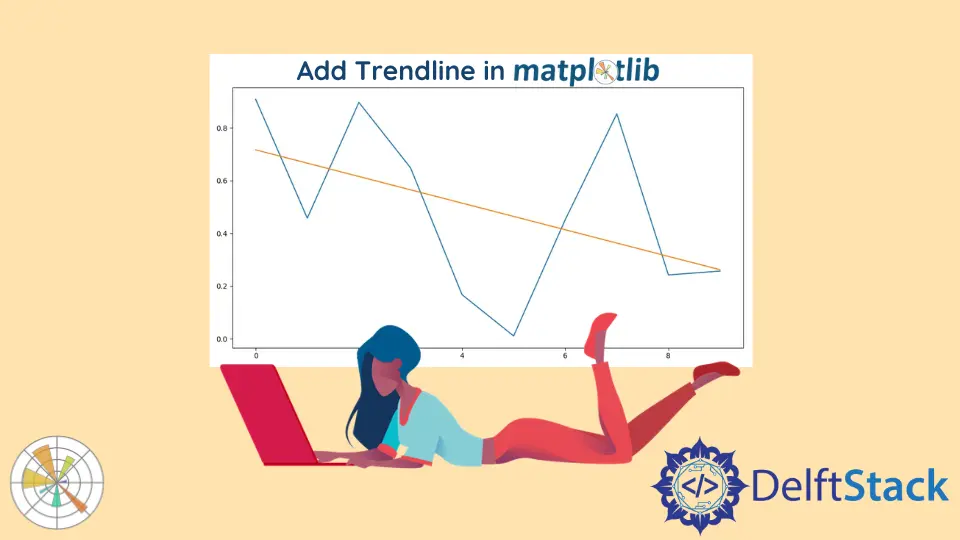
This tutorial will discuss adding a trendline to a plot in Matplotlib.
Generate Data to Plot in Matplotlib
Before working with plots, we need to set up our script to work with the library. We start by importing Matplotlib.
We load the randrange
function from the random
module to quickly generate some data. Hence, keep in mind that this will look different for you.
import numpy
from matplotlib import pyplot as plt
x = [x for x in range(0, 10)]
y = numpy.random.rand(10)
Add a Trendline With NumPy
in Python Matplotlib
The trendlines show whether the data is increasing or decreasing. For example, the Overall Temperatures on Earth may look fluctuating, but they are rising.
We calculate the trendline with NumPy
. To do that, we need the x- and y-axis.
Then we use the polyfit
and poly1d
functions of NumPy
. And finally, we plot the trendline.
# Plot the Data itself.
plt.plot(x, y)
# Calculate the Trendline
z = numpy.polyfit(x, y, 1)
p = numpy.poly1d(z)
# Display the Trendline
plt.plot(x, p(x))
Output:
Complete Code
import numpy
from matplotlib import pyplot as plt
x = [x for x in range(0, 10)]
y = numpy.random.rand(10)
# Plot the Data itself.
plt.plot(x, y)
# Calculate the Trendline
z = numpy.polyfit(x, y, 1)
p = numpy.poly1d(z)
# Display the Trendline
plt.plot(x, p(x))
plt.show()
Hi, my name is Maxim Maeder, I am a young programming enthusiast looking to have fun coding and teaching you some things about programming.
GitHub