How to Use Kronecker Delta in MATLAB
- What is the Kronecker Delta?
- Using Logical Indexing to Implement Kronecker Delta
- Using the Built-in Functions for Kronecker Delta
- Vectorized Implementation of Kronecker Delta
- Conclusion
- FAQ
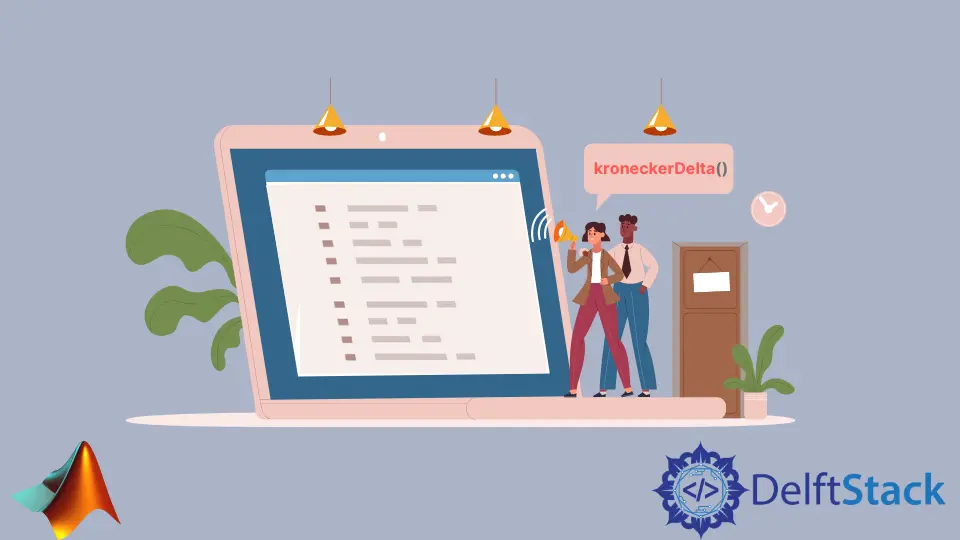
Understanding the Kronecker delta function is essential for various applications in mathematics, physics, and engineering. This mathematical function is particularly useful in linear algebra, signal processing, and systems theory.
In this tutorial, we will explore how to effectively use the Kronecker delta in MATLAB. We will walk you through the concept, provide practical examples, and demonstrate how to implement it in your MATLAB projects. By the end of this article, you will have a solid grasp of the Kronecker delta and be able to apply it in your own work.
What is the Kronecker Delta?
The Kronecker delta, denoted as δ(i, j), is a function of two variables, typically integers. It is defined as:
- δ(i, j) = 1 if i = j
- δ(i, j) = 0 if i ≠ j
This function is widely used in mathematical formulations and can simplify complex expressions in linear algebra. In MATLAB, implementing the Kronecker delta can be done using various methods, which we will discuss in detail.
Using Logical Indexing to Implement Kronecker Delta
One of the simplest ways to implement the Kronecker delta in MATLAB is through logical indexing. This method utilizes MATLAB’s ability to handle arrays and logical operations efficiently. Here’s how you can do it:
function delta = kronecker_delta(i, j)
delta = double(i == j);
end
% Example usage
result1 = kronecker_delta(3, 3);
result2 = kronecker_delta(2, 5);
Output:
result1 = 1
result2 = 0
In this example, we define a function kronecker_delta
that takes two inputs, i
and j
. Inside the function, we use the equality operator ==
to check if the two inputs are equal. The result is a logical value (true or false), which we convert to a double (1 or 0) to match the definition of the Kronecker delta. When we call the function with the values 3 and 3, it returns 1, indicating equality. Conversely, when we call it with 2 and 5, it returns 0, indicating inequality.
Using the Built-in Functions for Kronecker Delta
Another efficient way to implement the Kronecker delta in MATLAB is by leveraging built-in functions. MATLAB allows for array operations, making it easy to compute the Kronecker delta over arrays. Here’s an example:
function delta_matrix = kronecker_delta_matrix(n)
delta_matrix = zeros(n);
for i = 1:n
for j = 1:n
delta_matrix(i, j) = (i == j);
end
end
end
% Example usage
delta_3x3 = kronecker_delta_matrix(3);
Output:
delta_3x3 =
1 0 0
0 1 0
0 0 1
In this example, we create a function kronecker_delta_matrix
that generates a square matrix of size n
. We initialize a matrix of zeros and then loop through each element to assign 1 where the row index equals the column index, adhering to the definition of the Kronecker delta. The result is a diagonal matrix where all diagonal elements are 1, and all off-diagonal elements are 0, perfectly illustrating the Kronecker delta function.
Vectorized Implementation of Kronecker Delta
For those looking for a more MATLAB-esque way to implement the Kronecker delta, vectorization is the key. This method avoids explicit loops and takes advantage of MATLAB’s optimized array operations, leading to cleaner and often faster code. Here’s how you can implement it:
function delta_vector = kronecker_delta_vector(i, j)
delta_vector = (i == j);
end
% Example usage
result_vector1 = kronecker_delta_vector([1, 2, 3], [1, 1, 2]);
Output:
result_vector1 =
1 0 0
In this implementation, the function kronecker_delta_vector
takes two vectors as inputs. The expression (i == j)
automatically performs element-wise comparison, resulting in a logical array that indicates where the elements of the two vectors are equal. This approach is not only concise but also takes full advantage of MATLAB’s capabilities, making it a preferred method for many users.
Conclusion
In this tutorial, we explored the Kronecker delta function and its applications in MATLAB. We discussed various methods to implement the Kronecker delta, from basic logical indexing to vectorized operations. By understanding how to use the Kronecker delta in MATLAB, you can simplify your mathematical computations and enhance your programming skills. Whether you’re working on linear algebra problems or signal processing tasks, the Kronecker delta is a valuable tool in your MATLAB toolkit.
FAQ
-
What is the Kronecker delta used for?
The Kronecker delta is used in mathematics and engineering to simplify expressions and represent discrete functions. -
Can I use the Kronecker delta in other programming languages?
Yes, the Kronecker delta can be implemented in various programming languages, including Python, C++, and R. -
Is the Kronecker delta only applicable to integers?
While it is primarily defined for integers, the concept can be extended to other types of data in specific contexts. -
How do I visualize the Kronecker delta in MATLAB?
You can visualize the Kronecker delta by plotting the resulting matrix or using heatmaps to represent the values. -
Are there any built-in functions for Kronecker delta in MATLAB?
MATLAB does not have a specific built-in function for Kronecker delta, but you can easily create your own using the methods described above.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook