System of Linear Equation in MATLAB
- Understanding Systems of Linear Equations
-
Solving Linear Equations with the
solve()
Function -
Solving Linear Equations with the
linsolve()
Function - Conclusion
- FAQ
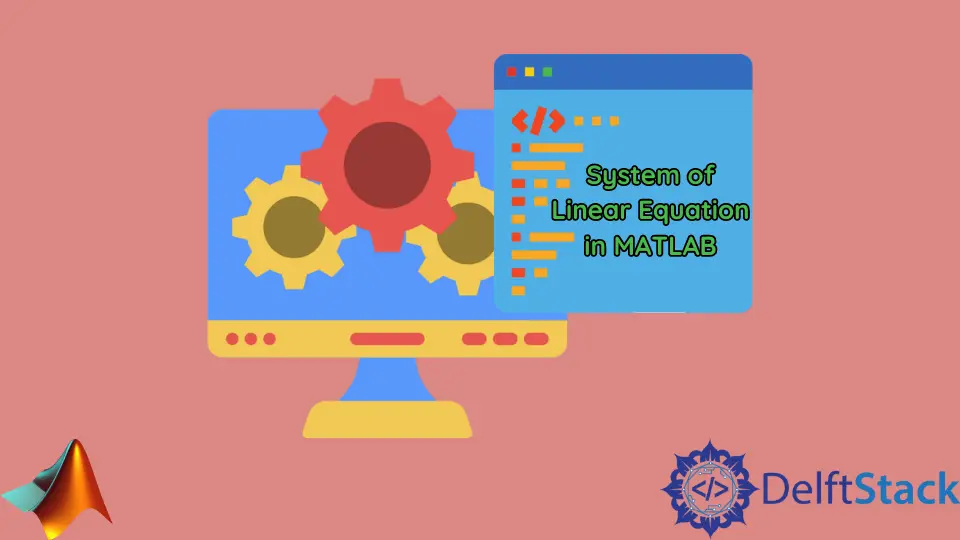
Solving systems of linear equations is a fundamental task in various fields of engineering and science. MATLAB, with its powerful computational capabilities, provides efficient functions to tackle these equations.
In this tutorial, we will delve into two primary functions: solve()
and linsolve()
. Whether you’re dealing with a small set of equations or a larger system, understanding how to use these functions will enhance your problem-solving toolkit. We will explore the syntax, provide clear examples, and discuss the nuances of each method to ensure you can effectively apply them in your projects. Let’s get started!
Understanding Systems of Linear Equations
A system of linear equations consists of multiple linear equations involving the same set of variables. For example, consider the equations:
- 2x + 3y = 5
- 4x - y = 1
The goal is to find values for x and y that satisfy both equations simultaneously. MATLAB provides robust functions to solve such systems efficiently.
Solving Linear Equations with the solve()
Function
The solve()
function in MATLAB is part of the Symbolic Math Toolbox and is particularly useful for solving equations symbolically. This function can handle both linear and nonlinear equations, making it versatile for various applications.
To solve a system of linear equations using solve()
, you can follow these steps:
- Define the symbolic variables.
- Set up the equations.
- Use the
solve()
function to find the solutions.
Here’s how that looks in code:
syms x y
eq1 = 2*x + 3*y == 5;
eq2 = 4*x - y == 1;
solution = solve([eq1, eq2], [x, y]);
Output:
solution.x = 1
solution.y = 1
In this example, we first declare x
and y
as symbolic variables. The equations are defined using the equality operator (==
). When we call solve()
, we provide the equations and the variables we want to solve for. The function returns a structure containing the solutions for x
and y
, which in this case are both equal to 1.
Using solve()
is particularly beneficial when you need exact solutions, especially in theoretical contexts where precision is crucial. However, it may not be the fastest method for large systems due to its symbolic nature.
Solving Linear Equations with the linsolve()
Function
For numerical solutions, especially with larger systems, the linsolve()
function is often the go-to choice in MATLAB. This function is designed specifically for solving linear equations represented in matrix form, making it both efficient and straightforward.
To utilize linsolve()
, you need to express your system of equations in matrix form, Ax = b, where A is the coefficient matrix, x is the vector of variables, and b is the constants vector.
Here’s a practical example:
A = [2, 3; 4, -1];
b = [5; 1];
solution = linsolve(A, b);
Output:
solution(1) = 1
solution(2) = 1
In this code, we define the coefficient matrix A
and the constants vector b
. The linsolve()
function then computes the solution vector directly. The output shows that both variables x
and y
equal 1, just as we found with the solve()
function.
Using linsolve()
is advantageous when dealing with large systems, as it leverages optimized algorithms for numerical computations. It is generally faster and consumes less memory compared to symbolic methods, making it ideal for practical applications in engineering and data analysis.
Conclusion
In summary, solving systems of linear equations in MATLAB can be accomplished through various methods, with solve()
and linsolve()
being the most prominent. The choice between these functions largely depends on the nature of your equations—whether you need symbolic or numerical solutions. By mastering these techniques, you can effectively tackle a wide range of problems in mathematics, engineering, and beyond. With practice, these tools will become invaluable in your MATLAB arsenal.
FAQ
-
What is the difference between solve() and linsolve() in MATLAB?
solve() is used for symbolic solutions and can handle both linear and nonlinear equations, while linsolve() is optimized for numerical solutions of linear equations. -
Can I use solve() for large systems of equations?
While you can use solve() for large systems, it may not be efficient. linsolve() is recommended for better performance with large datasets. -
Is it necessary to have the Symbolic Math Toolbox to use solve()?
Yes, thesolve()
function is part of the Symbolic Math Toolbox, which needs to be installed separately in MATLAB. -
How can I visualize the solutions of the equations in MATLAB?
You can use plotting functions like plot() or fplot() to visualize the equations and their intersections, which represent the solutions.
- Are there any limitations to using linsolve()?
linsolve() requires the coefficient matrix to be square and non-singular. If these conditions are not met, it may not provide a solution.