How to Convert RGB to Gray Scale in Matlab
- Convert an RGB Image to Grayscale Without Using Any Functions in MATLAB
-
Convert an RGB Image to Gray Scale Using the
rgb2gray()
Function in MATLAB
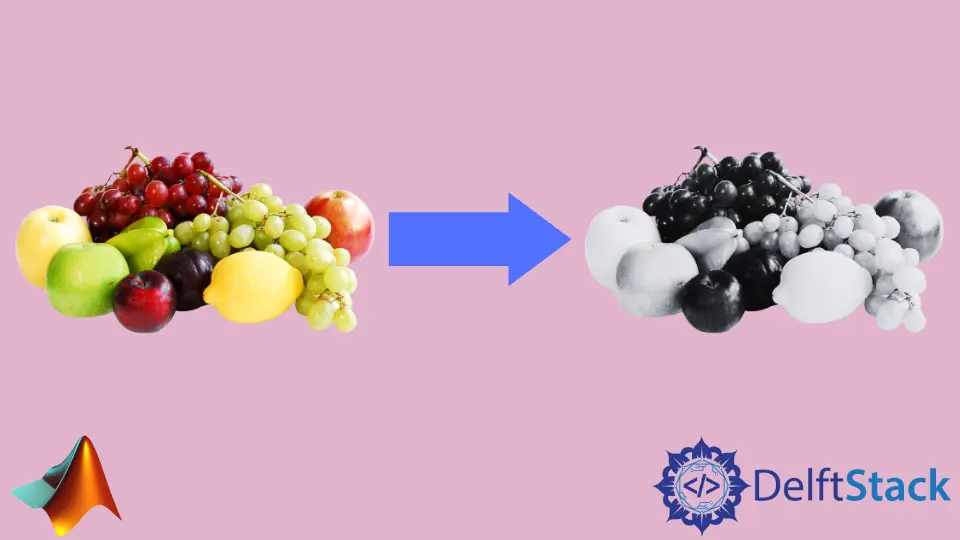
This tutorial will discuss how to convert an RGB image to grayscale manually and using the rgb2gray()
function in MATLAB.
Convert an RGB Image to Grayscale Without Using Any Functions in MATLAB
You can convert an RGB image to grayscale without using any functions in MATLAB. MATLAB reads an image and returns a matrix containing values from 0 to 255, which are actually the color of each pixel present in the image. You just need to convert the colors to gray. For example, let’s read an RGB image and convert it into grayscale without using any function in MATLAB. See the code below.
input_image = imread('peppers.png');
input_image = im2double(input_image);
gray_image = .299*input_image(:,:,1) + .587*input_image(:,:,2) + .114*input_image(:,:,3);
imshowpair(input_image,gray_image,'montage');
Output:
In the above code, we used an already present image of peppers in MATLAB and converted it into grayscale without using any functions. In the above figure, the left image is the input RGB image, and the right image is the result of the conversion. We used imshowpair()
to show images side by side for a better understanding of the conversion.
Convert an RGB Image to Gray Scale Using the rgb2gray()
Function in MATLAB
You can convert an RGB image to grayscale using the rgb2gray()
function in MATLAB. For example, let’s read an RGB image and convert it into grayscale using the rgb2gray()
function in MATLAB. See the code below.
input_image = imread('peppers.png');
gray_image = rgb2gray(input_image);
imshowpair(input_image,gray_image,'montage');
Output:
In the above code, we used an already present image of peppers in MATLAB and converted it into grayscale using the rgb2gray()
function. In the above figure, the left image is the input RGB image, and the right image is the result of the conversion. We used imshowpair()
to show images side by side for a better understanding of the conversion.