MATLAB Recursive Function
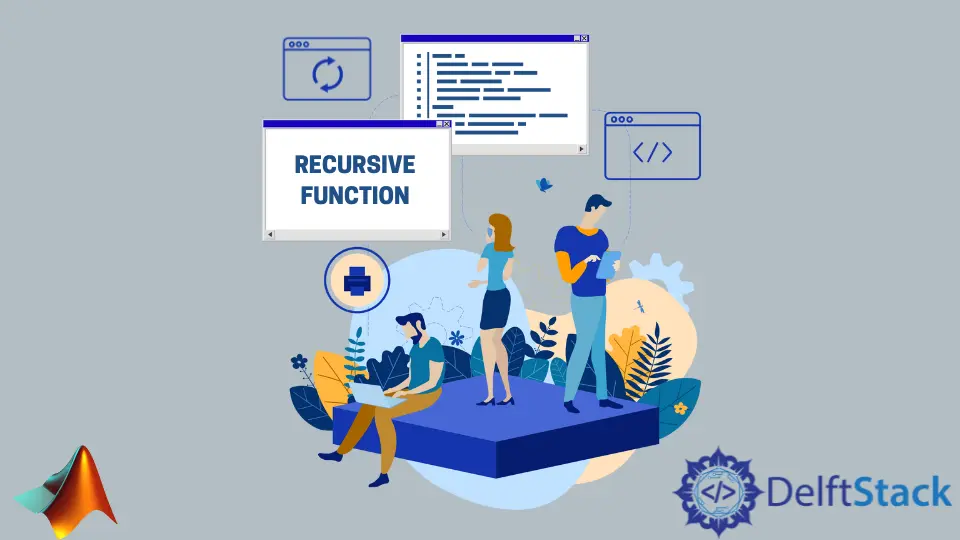
This tutorial will discuss how to define a recursive function in MATLAB.
Recursive Function in MATLAB
A function that calls itself during its execution is called a recursive function. The recursive function keeps on calling itself until certain conditions are achieved. For example, let’s define a recursive function to find the factorial of a given number. See the code below.
myFactorial = factorial(5)
function output=factorial(input)
if (input<=0)
output=1;
else
output=input*factorial(input-1);
end
end
Output:
myFactorial =
120
In the above code, we defined a recursive factorial function that will find the factorial of a given number. This function will call itself until the input is less than or equal to zero; after that, the result will be returned. As you can see in the output, we calculated the factorial of 5, which is 120. You can define your own recursive function as long as you know the condition you want to achieve.