How to Print Output in Command Window in Matlab
-
Use the
disp()
Method to Print Output in Command Window in Matlab -
Use the
fprintf()
Method to Print Output in Command Window in Matlab
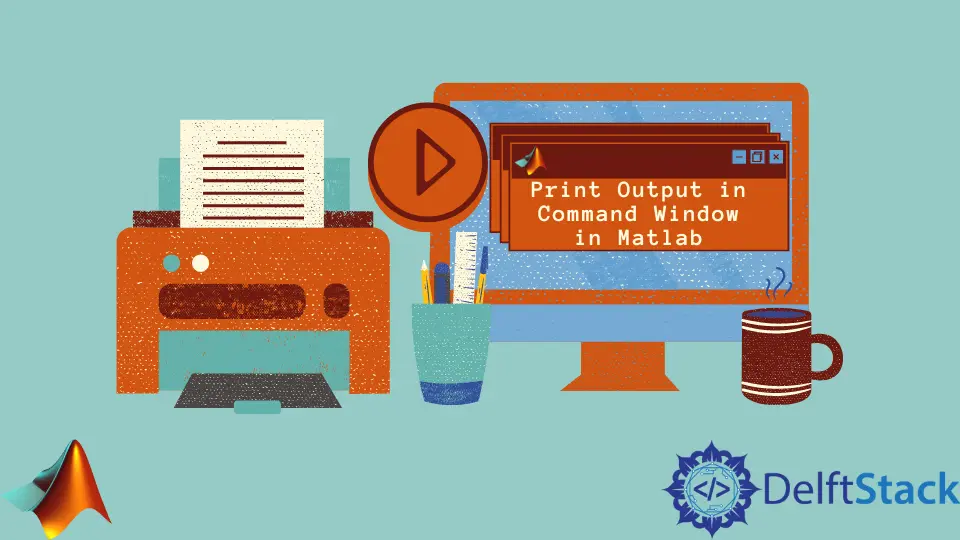
This tutorial demonstrates how to print output in the command window using Matlab.
There are different ways to print outputs in the command window in Matlab. We can use the fprintf()
or disp()
methods.
Use the disp()
Method to Print Output in Command Window in Matlab
The disp()
method displays the value of a variable in Matlab. For instance, disp(a)
will display the value of the variable a
without the variable name.
If you want to display the value of a variable with a name, you can display a =
first and then the variable.
The disp()
method can display any variable. If the variables, like arrays, are empty, the disp()
will not print anything.
Example:
A = [5 10 15
20 25 30
35 40 45 ];
B = 'Hello! This is Delftstack.com';
C = 100;
disp('A = ')
disp(A)
disp('B = ')
disp(B)
disp('C = ')
disp(C)
The code above will display the values of the variables A
(array), B
(string), and C
(integer).
Output:
>> matlabprintcommand
A =
5 10 15
20 25 30
35 40 45
B =
Hello! This is Delftstack.com
C =
100
Use the fprintf()
Method to Print Output in Command Window in Matlab
The fprintf()
method in Matlab prints the values in the command window with a specific format. For example, fprintf(fileID,formatSpec,A1,...,An)
will apply the formatSpec
to all elements of the array from A1
to An
in the column order.
It will also write the data to a text file using the fileID
.
Example:
A1 = 'Jack';
A2 = 'John';
A3 = 'Mike';
A4 = 'Michelle';
formatSpec = 'The employee name is %s\n';
fprintf(formatSpec, A1, A2, A3, A4);
The code above will display the values according to the given format specifications.
Output:
>> matlabprintcommand
The employee name is Jack
The employee name is John
The employee name is Mike
The employee name is Michelle
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook