Piecewise Function in MATLAB
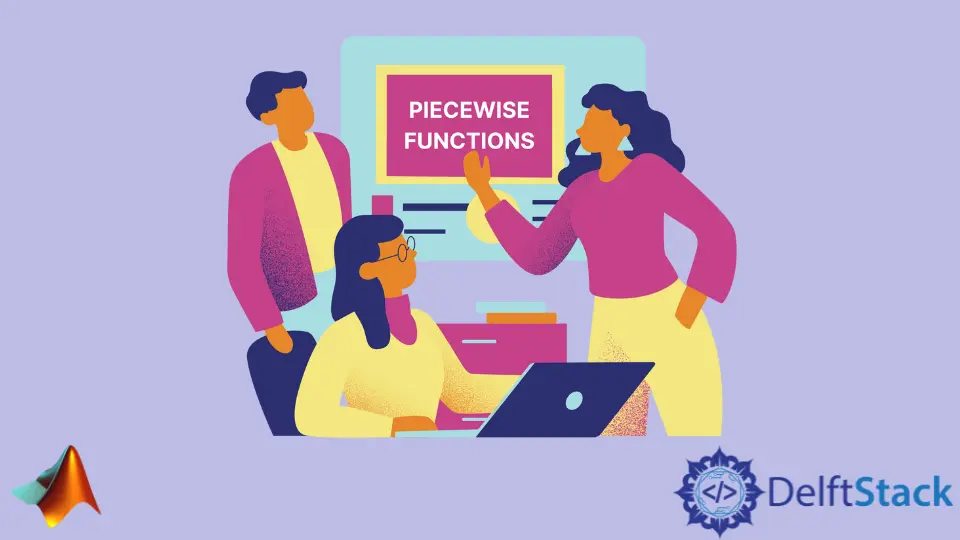
This tutorial will discuss how to define a piecewise function or expression using the piecewise()
function in MATLAB.
Define a Piecewise Function or Expression Using the piecewise()
Function in MATLAB
To define a piecewise function or expression in MATLAB, you can use the piecewise()
function. This function returns a function or expression which contains a piecewise function or expression. To define a piecewise function, you have to put the condition and its value inside the piecewise()
function and then the second condition and its value, and so on. You can also set the value which will be true when no condition is true. For example, let’s define a simple piecewise function. See the code below.
syms y(x)
y(x) = piecewise(x<0, -2, x>0, 2, 1);
y(-3)
Output:
ans =
-2
In the above code, we defined a piecewise function whose value is -2 if x is less than zero and 2 if x is greater than zero, and if none of the conditions is true, its value will be 1. The third line is used to test the function, and you can see in the output, the function is performing correctly. Check this link for more details about the piecewise()
function.