How to Get Negative of an Image in MATLAB
- Understanding Image Negation
- Method 1: Using MATLAB’s Built-in Functions
- Method 2: Manual Pixel Manipulation
- Method 3: Using Image Processing Toolbox
- Conclusion
- FAQ
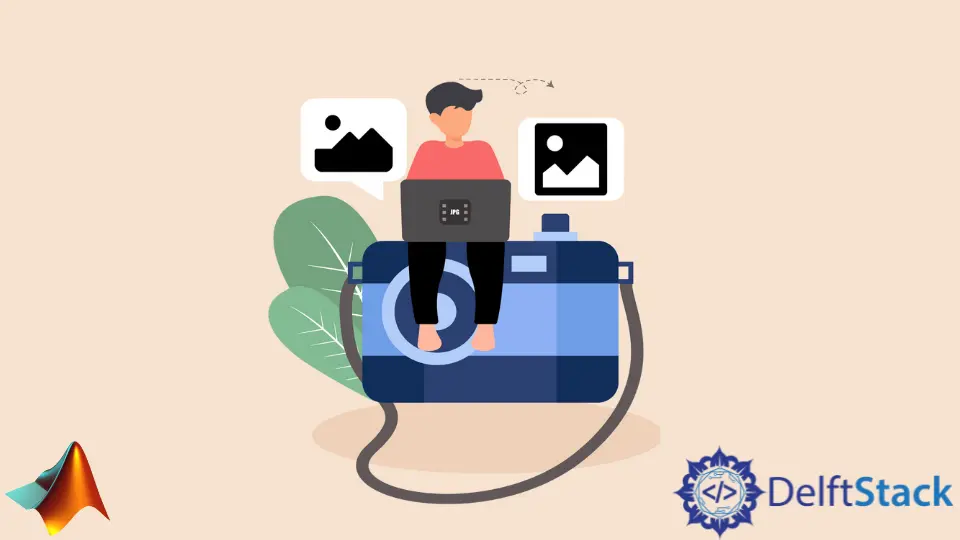
Creating the negative of an image is a fascinating process that can enhance your understanding of image processing in MATLAB. By changing the intensity levels of the pixels, you can transform a standard image into its negative counterpart. This technique is not just an artistic endeavor; it serves various practical applications in image analysis and computer vision.
In this article, we will explore how to achieve this effect using MATLAB, providing clear examples and explanations along the way. Whether you’re a student, a professional, or just a curious mind, this guide will help you master the art of image manipulation in MATLAB.
Understanding Image Negation
Before diving into the practical aspects, it’s essential to grasp what creating an image negative entails. In simple terms, the negative of an image is produced by inverting the pixel values. If a pixel has a value of 255 (white), its negative will be 0 (black), and vice versa. This inversion can be achieved by subtracting the pixel values from the maximum intensity level, which is typically 255 for an 8-bit image.
Method 1: Using MATLAB’s Built-in Functions
MATLAB provides a straightforward way to create an image negative using its built-in functions. Here’s how you can do it:
% Read the image
img = imread('your_image.jpg');
% Convert to grayscale if it's a colored image
if size(img, 3) == 3
img = rgb2gray(img);
end
% Create the negative image
negative_img = 255 - img;
% Display the original and negative images
subplot(1, 2, 1);
imshow(img);
title('Original Image');
subplot(1, 2, 2);
imshow(negative_img);
title('Negative Image');
Output:
This code begins by reading an image file and checking if it is colored. If it is, the image is converted to grayscale to simplify the process. The negative image is created by subtracting the pixel values from 255, effectively inverting the colors. Finally, both the original and negative images are displayed side by side for comparison. This approach is efficient and leverages MATLAB’s powerful image processing capabilities.
Method 2: Manual Pixel Manipulation
If you prefer a more hands-on approach, you can manually manipulate the pixel values to create an image negative. This method gives you a deeper insight into how pixel values work in images.
% Read the image
img = imread('your_image.jpg');
% Convert to grayscale if it's a colored image
if size(img, 3) == 3
img = rgb2gray(img);
end
% Initialize the negative image
[rows, cols] = size(img);
negative_img = zeros(rows, cols, 'uint8');
% Loop through each pixel
for i = 1:rows
for j = 1:cols
negative_img(i, j) = 255 - img(i, j);
end
end
% Display the original and negative images
subplot(1, 2, 1);
imshow(img);
title('Original Image');
subplot(1, 2, 2);
imshow(negative_img);
title('Negative Image');
Output:
In this method, we first read and convert the image to grayscale, just like in the previous example. The key difference lies in how we create the negative image. Here, we initialize a new matrix for the negative image and loop through each pixel, manually inverting the values. This method not only provides the same result but also enhances your understanding of how images are represented in MATLAB.
Method 3: Using Image Processing Toolbox
MATLAB’s Image Processing Toolbox offers advanced functionality for image manipulation. Using this toolbox, you can create an image negative efficiently.
% Read the image
img = imread('your_image.jpg');
% Convert to grayscale if it's a colored image
if size(img, 3) == 3
img = rgb2gray(img);
end
% Create the negative image using imcomplement
negative_img = imcomplement(img);
% Display the original and negative images
subplot(1, 2, 1);
imshow(img);
title('Original Image');
subplot(1, 2, 2);
imshow(negative_img);
title('Negative Image');
Output:
In this method, we utilize the imcomplement
function from the Image Processing Toolbox, which simplifies the task of creating an image negative. After reading and converting the image, we call imcomplement
to directly obtain the negative image. This approach is not only concise but also highly efficient, making it a great option for those familiar with MATLAB’s toolbox functions.
Conclusion
Creating the negative of an image in MATLAB is a valuable skill that can enhance your image processing toolkit. Whether you choose to use built-in functions, manually manipulate pixel values, or leverage the Image Processing Toolbox, each method provides a unique perspective on how images can be transformed. As you experiment with these techniques, you’ll gain a deeper understanding of image manipulation and its applications in various fields. So, grab your MATLAB environment and start creating stunning image negatives today!
FAQ
-
What is the purpose of creating a negative image?
Creating a negative image can help in analyzing features, enhancing contrast, and improving visibility in certain applications. -
Can I use color images to create negatives in MATLAB?
Yes, you can create negatives of color images by converting them to grayscale first or by inverting each color channel individually.
-
Is the Image Processing Toolbox necessary for creating image negatives?
No, you can create image negatives using basic MATLAB functions without the Image Processing Toolbox, although the toolbox offers more advanced features. -
What file formats can I use with imread in MATLAB?
You can use various formats such as JPG, PNG, BMP, and TIFF with the imread function in MATLAB. -
How can I save the negative image after creating it?
You can use the imwrite function in MATLAB to save the negative image to your desired format.