MATLAB Ternary Operator
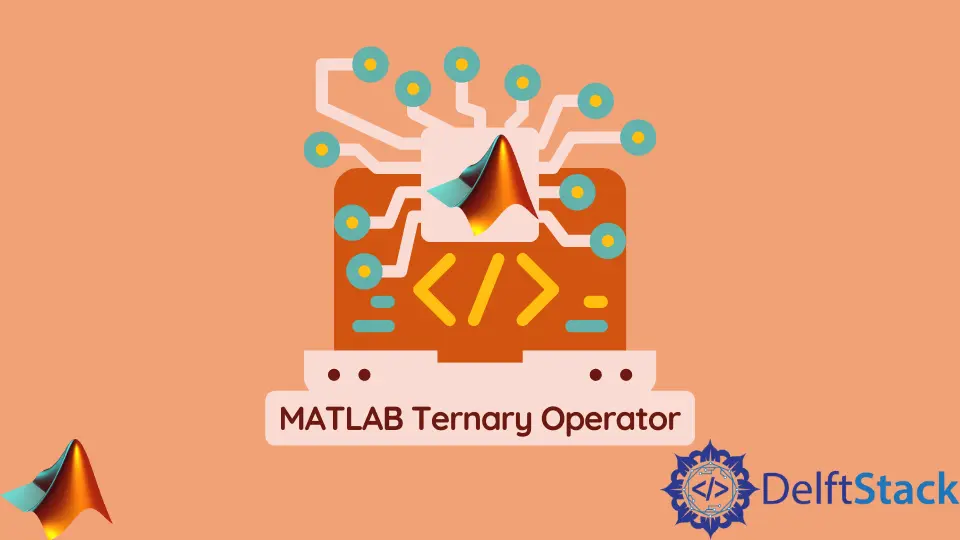
In this tutorial, we will discuss how we can use the ternary operator in MATLAB.
Ternary Operator in MATLAB
MATLAB does not have the ternary operator, so you have to use the if
statement. The ternary operator in some languages is used to define the else
statement. See the below code.
MyVariable = (condition) ? statement1 : statement2;
If the condition is true, the first statement, statement1
, will be executed, and if the condition is false, then the second statement, statement2
, will be executed. But this functionality is not supported by MATLAB. Instead, you have to use the if
statement to get the same result as the above code. See the below code.
if(condition)
statement1
else
statement2
end
In the above code, we have performed the same function as performed by the ternary operator. If the condition is true, the first statement, statement1
, will be executed, and if the condition is false, then the second statement, statement2
, will be executed.