How to Get Random Permutation Using MATLAB
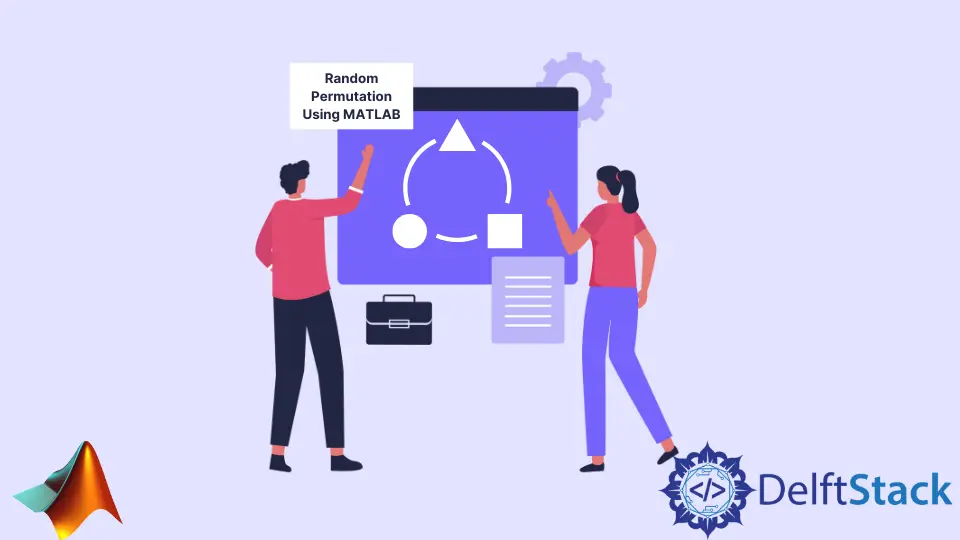
This tutorial will discuss generating vectors containing a random permutation of integers using the randperm()
function in MATLAB.
Generate Vectors Containing Random Permutation of Integers Using the randperm()
Function in MATLAB
We can use MATLAB’s built-in function randperm()
to generate vectors containing a random permutation of integers. We can define the largest integer in the sampling interval in the randperm()
function, and the smallest integer in the sampling interval is one by default.
For example, let’s generate six integers between the range 1 to 6 using the randperm()
function. See the code below.
v = randperm(6)
Output:
v =
3 5 6 1 4 2
The output will change if we rerun this code because these values are random. We can define the number of random permutation integers using the second argument of the randperm()
function.
We can only define a number less than or equal to the first argument because the generated numbers are unique and cannot be repeated. If we define a number greater than the first argument, MATLAB will show an error.
For example, let’s generate two random permutation numbers between 1 and 6 using the randperm()
function. See the code below.
v = randperm(6,2)
Output:
v =
1 3
Only two numbers in the output will change if we rerun the code because the generated numbers are random. We can also save the state of a random number generator using the rng
function.
The previously saved state can be used to generate the same sequence of integers. For example, let’s generate two vectors with the same sequence using the randperm()
and rng
functions.
See the code below.
s = rng;
v1 = randperm(6,2)
rng(s)
v2 = randperm(6,2)
Output:
v1 =
1 2
v2 =
1 2
In the output, both vectors v1 and v2 contain the same elements.
If we rerun the code, the output will change, but the elements of both vectors will remain unchanged. If we don’t use the previously-stored state, the vectors will have different elements.