MATLAB Optional Arguments
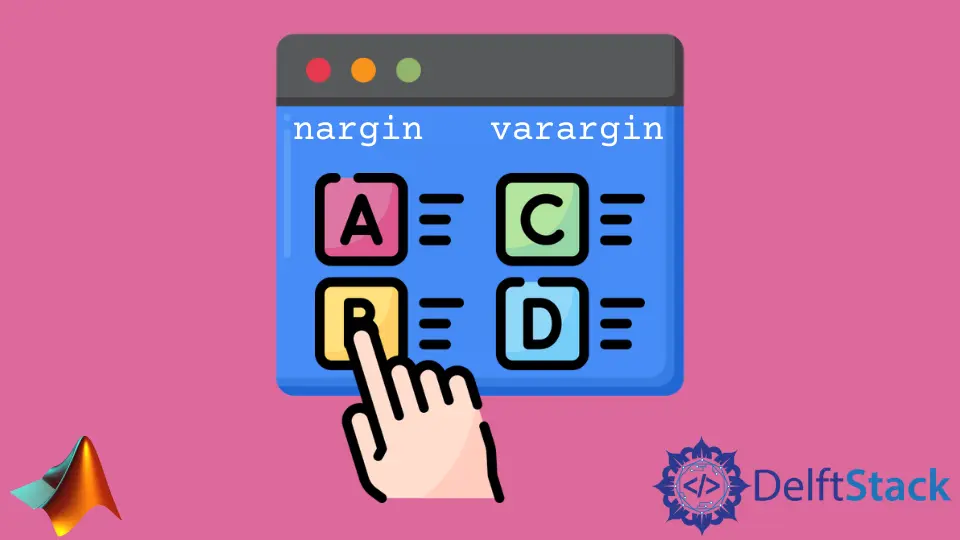
In this tutorial, we will discuss how to set the optional arguments of a function by using the nargin
and varargin
properties in MATLAB.
Set the Optional Arguments of a Function by Using the varargin
and nargin
Property in MATLAB
You can give any number of inputs to a function using the varargin
property inside the brackets of the function name, and you can check the number of inputs passed to the function using the nargin
property. For example, let’s create a function that accepts any number of inputs using the varargin
property and then get the number of inputs using the nargin
property. See the code below.
Number_of_inputs = sample(2,1,1)
function output = sample(varargin)
output = nargin;
end
Output:
Number_of_inputs =
3
As you can see, we passed three inputs in the function, which returns the total number of inputs. The varargin
contains an array of the inputs which you can use according to your need. For example, let’s get the number of inputs and then print those inputs using a for
loop. See the code below.
Number_of_inputs = sample(2,'Hello',[1,2,3]);
function output = sample(varargin)
output = nargin;
for i=1:nargin
disp(varargin{i})
end
end
Output:
2
Hello
1 2 3
As you can see in the output, the inputs have been displayed. So you can use the index to get a specific input saved in the varargin
cell array and then use them according to your requirements. All of these inputs are optional, but if you want to add some inputs that are mandatory, you can add them before the varargin
. Note that you have to add the mandatory inputs during the function call; otherwise, the function will give an error. For example, let’s add two mandatory arguments and then add them to get the output, and the optional arguments will be displayed. See the code below.
sum = sample(2,3,[1,2,3],'Hello')
function output = sample(x,y,varargin)
output = x+y;
for i=1:nargin-2
disp(varargin{i})
end
end
Output:
1 2 3
Hello
sum =
5
As you can see in the output, the first two inputs have been added to get the sum, and the optional arguments are displayed. Note that we have subtracted two from nargin
because nargin
gives the total number of inputs, not the inputs present in the varargin
cell array. Check this link to get more information about the varargin
property.