How to Return Multiple Values From a Matlab Function
Ammar Ali
Feb 02, 2024
MATLAB
MATLAB Function
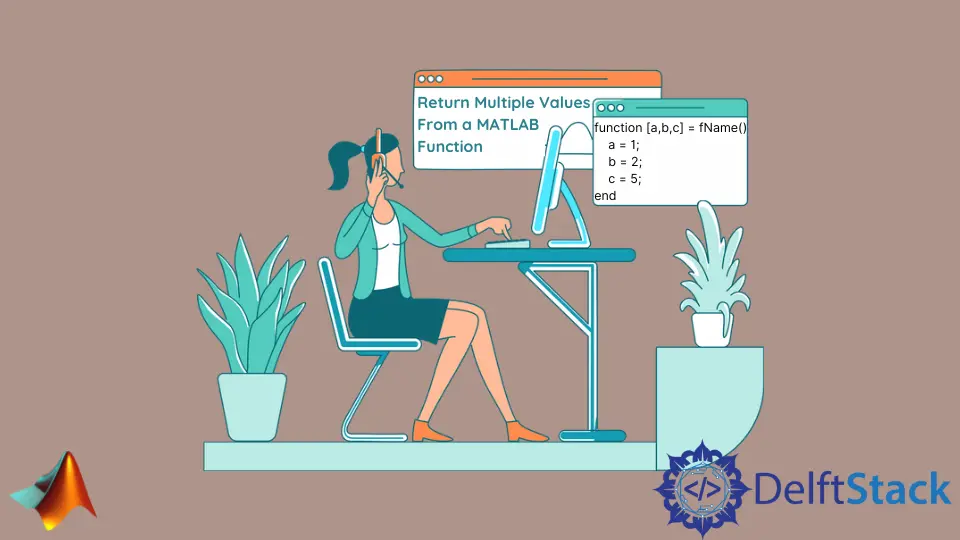
This tutorial will discuss how to return multiple values from a function using the box brackets in MATLAB.
Return Multiple Values From a Function Using the Box Brackets in MATLAB
If you want to return multiple values from a function, you have to define all of them inside a box bracket separated by a comma and assign them the required output within the function bounds. For example, let’s create a function and return three values. See the code below.
[a,b,c] = fName()
function [a,b,c] = fName()
a = 1;
b = 2;
c = 5;
end
Output:
a =
1
b =
2
c =
5
As you can see in the output, three values have been returned by the function.
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Author: Ammar Ali