MATLAB Max Index
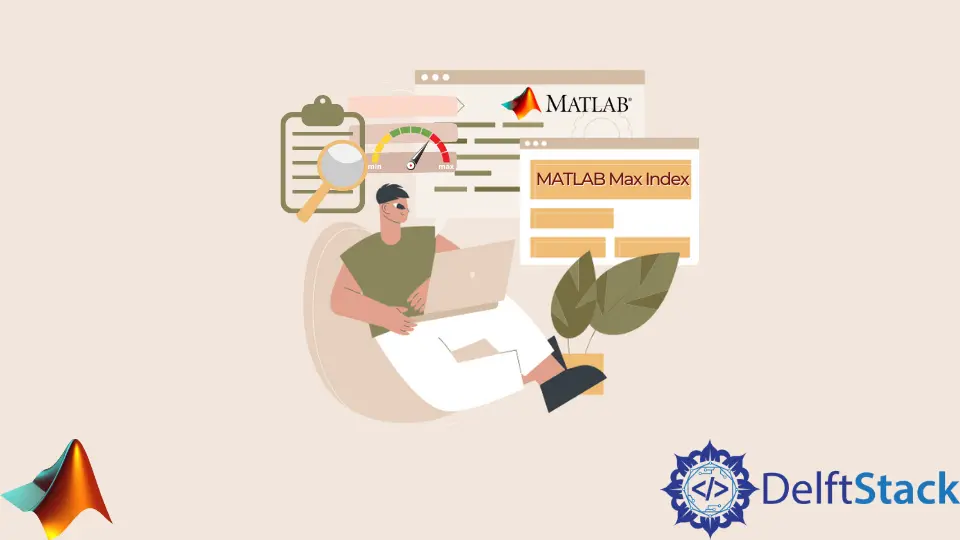
In this tutorial, we will discuss how to find the maximum value from an array and its index using the max()
function in MATLAB.
Find Maximum Value and Its Index Using the max()
Function in MATLAB
The max()
function in MATLAB gets the maximum value from a given array or matrix. In the case of an array, it will return the value of the maximum value present in that array along with its index. In the case of a matrix, it will return the maximum values from each column of the matrix in the form of a vector containing maximum values present in all the columns. For example, let’s say we want to get the maximum value and its index from a vector or array of integers. See the below code.
myArray = [1 2 3 4 5]
[mValue , vIndex] = max(nyArray)
The maximum value will be stored in mValue
, and its index will be stored in vIndex
. Now let’s finds the maximum value and its index from each column of the matrix. See the below code.
myMatrix = [1 2 3; 4 5 6]
[mValues , vIndices] = max(myMatrix)
In the above code, we used a matrix that has two rows and three columns. The result will contain three maximum values, and three indices as the number of columns in the matrix is three. This function can also be used to replace the specific values of a matrix with a scaler. For example, consider we want to replace every value in the matrix which is less than a specific scaler. See the below code.
myMatrix = [1 2 4; 4 6 8]
aScaler = 3;
newMatrix = max(myMatrix,aScaler)
In the above code, we are replacing each value of the matrix myMatrix
, which is less than the scaler aScaler
, with the value stored in aScaler
.