Masking in MATLAB
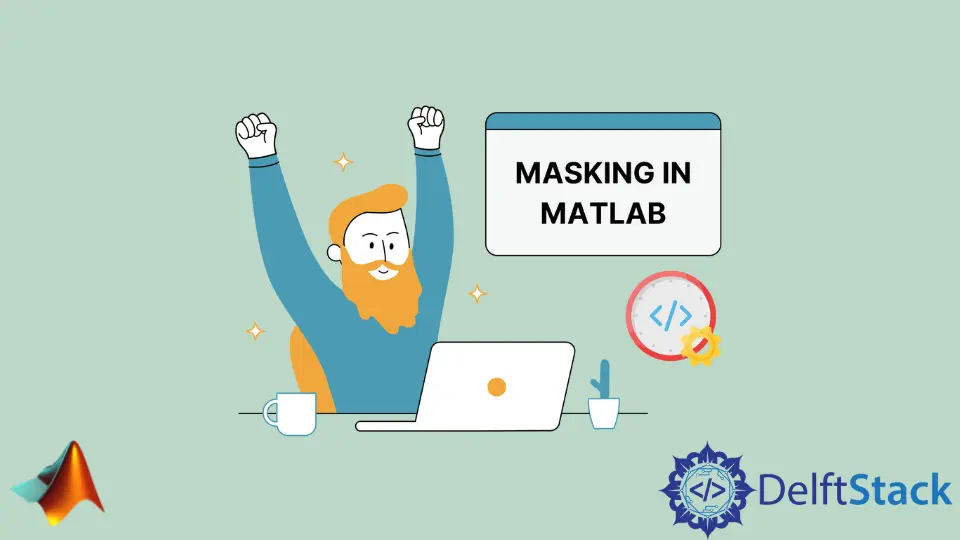
This tutorial demonstrates the concept of masking in MATLAB.
Masking in MATLAB
A Mask
is considered a custom interface for a block that is used to hide the content of that block and make it appear as an atomic block with the given parameter dialog box and the block’s icon.
The mask will encapsulate the logic of the block and will provide controlled access to the block data; it also simplifies the model’s graphical appearance.
A mask definition will be created and saved with the block whenever we mask a block. A mask will not change the characteristics of a block; it will only change the interface of the block.
We can provide access to one or more underlying block parameters by defining the mask parameter on the mask. Masking can be used for the following reasons.
- To display an icon on the block.
- When a customized dialog box is needed for the block.
- Initializing parameters using MATLAB code.
- When a customized description is needed which is specific to the masked block.
We can also generate logical masking using MATLAB code besides the Mask editor. Here is an example.
%random structure with size(x) = size(y) = size(z)
Demo_Struct.x = round(rand(3,7)*2-1);
Demo_Struct.y = round(rand(3,7)*2-1);
Demo_Struct.z = round(rand(3,7)*2-1);
%logical mask
mask = Demo_Struct.x>=0;
%applying the mask to each field
New_Struct = structfun(@(p) p(mask),Demo_Struct,'UniformOutput',0)
The code above tries to create a mask from one of the fields of a struct data type and then applies it to the whole struct. The output will show that the mask is applied to the whole struct.
New_Struct =
x: [15x1 double]
y: [15x1 double]
z: [15x1 double]
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook