MATLAB Index Exceeds Matrix Dimensions
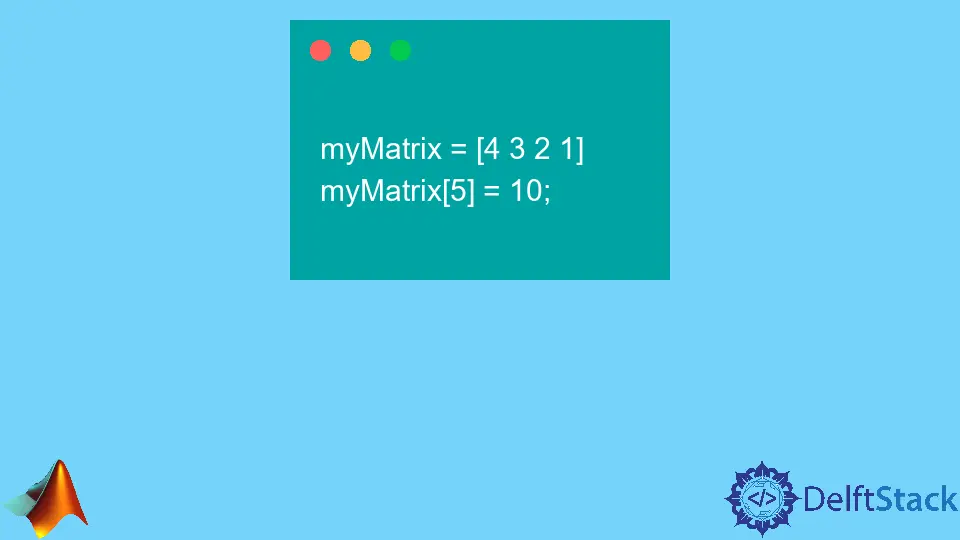
In this tutorial, we will discuss how to solve the index exceeds matrix dimensions problem in MATLAB.
Index Exceeds Matrix Dimensions Problem in MATLAB
In MATLAB, every array or matrix element is stored on a specific index which starts from 1 and increases as the number of elements increases in that array or matrix. To get an element or to replace an element in an array or matrix, we use the index of that element. If an array has ten elements in it, their indices range will vary from 1 to 10, respectively.
If we try to get or replace an element using an index that is 11 or larger, which is not in the range of the indices, then MATLAB will give us an error saying the index exceeds matrix dimensions. So make sure to use the index value which is inside the indices range. You can use the size()
function to check the size of your array or matrix before using an index value. For example, see the below code.
myMatrix = [4 3 2 1]
myMatrix[5] = 10;
In the above code, we are saving a value of 10 at the index value of 5 in the matrix myMatrix
. But as you can see, the number of indices present in the myMatrix
is only four. That means we will get an error of index exceeding matrix dimensions. To solve this problem, we have to save the value at an index inside the indices range which is 1 to 4. See the corrected code below.
myMatrix = [4 3 2 1]
myMatrix[4] = 10;
In the above code, we are saving a value of 10
at the index value of 4
in the matrix myMatrix
. As you can see, the index is inside the indices range, so the value 1
in the matrix myMatrix
will be replaced with the value 10
.