MATLAB Global Variables
- What Are Global Variables in MATLAB?
- Declaring Global Variables
- Best Practices for Using Global Variables
- Conclusion
- FAQ
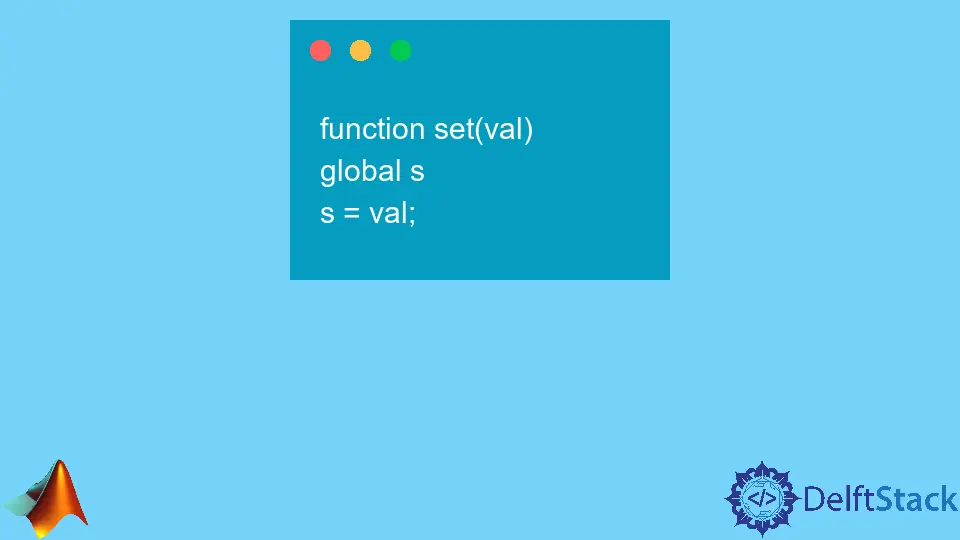
In the world of programming, especially in MATLAB, managing data efficiently is crucial for building robust applications. One effective way to share variables between multiple functions is by using global variables. By declaring variables as global, you enable different functions to access and modify the same data without needing to pass them explicitly. This can simplify your code and enhance performance, especially when dealing with large datasets or complex calculations.
In this article, we will explore the concept of global variables in MATLAB, how to declare them, and best practices for their use. Whether you’re a beginner or an experienced programmer, understanding this feature can significantly improve your coding efficiency.
What Are Global Variables in MATLAB?
Global variables in MATLAB are special types of variables that can be accessed by any function within the same workspace. Unlike local variables, which are confined to the function in which they are declared, global variables exist in a shared space. This means that when you modify a global variable in one function, the change is reflected in all other functions that access it.
To declare a global variable in MATLAB, you simply use the global
keyword followed by the variable name. It’s essential to declare the variable as global in every function that needs to access it. This approach can be particularly useful in scenarios where multiple functions need to read from or write to the same data.
Here’s a simple example:
function firstFunction
global myVar
myVar = 10;
end
function secondFunction
global myVar
disp(myVar)
end
In this example, calling firstFunction
sets myVar
to 10, and when secondFunction
is called, it displays the value of myVar
. Both functions can access the same global variable seamlessly.
Output:
10
The use of global variables can streamline your code and reduce the need for passing arguments between functions. However, it’s essential to use them judiciously, as over-reliance on global variables can lead to code that is difficult to debug and maintain.
Declaring Global Variables
To effectively use global variables in MATLAB, you need to understand how to declare them properly. The declaration process is straightforward but requires attention to detail. You must declare the variable as global in each function where it is used.
Here’s a step-by-step breakdown of how to declare and use global variables:
- Declare the variable: Use the
global
keyword followed by the variable name at the beginning of each function that will access the variable. - Assign a value: In one of the functions, assign a value to the global variable.
- Access the variable: In other functions, simply declare the variable as global again to access its value.
Here’s a more comprehensive example:
function mainFunction
global sharedVar
sharedVar = 5;
displayValue();
modifyValue();
displayValue();
end
function displayValue
global sharedVar
disp(sharedVar)
end
function modifyValue
global sharedVar
sharedVar = sharedVar + 5;
end
In this example, mainFunction
initializes sharedVar
to 5, then calls displayValue
, which shows the current value. The modifyValue
function increments sharedVar
by 5, and when displayValue
is called again, it reflects the updated value.
Output:
5
10
By following these steps, you can effectively manage data across multiple functions in MATLAB, enhancing the modularity and clarity of your code.
Best Practices for Using Global Variables
While global variables can simplify data sharing between functions, they come with their own set of challenges. Here are some best practices to ensure that you use global variables effectively and maintain the integrity of your code:
- Limit usage: Use global variables sparingly. Overusing them can lead to code that is difficult to read and maintain.
- Document your code: Clearly document where and why you are using global variables. This helps others (and your future self) understand the rationale behind your design choices.
- Avoid naming conflicts: Choose unique names for your global variables to avoid conflicts with local variables or other global variables in your code.
- Use clear naming conventions: Adopt a consistent naming convention that indicates a variable is global, making it easier to identify at a glance.
- Consider alternatives: In many cases, passing variables as function arguments may be a better design choice. This approach enhances code clarity and reduces dependencies between functions.
Here’s an example that follows these best practices:
function mainProgram
global configVar
configVar = 'Initial Config';
updateConfig();
showConfig();
end
function updateConfig
global configVar
configVar = 'Updated Config';
end
function showConfig
global configVar
disp(configVar)
end
Output:
Updated Config
In this example, configVar
is used as a global variable to store configuration data. By following the best practices outlined, you can ensure that your use of global variables remains manageable and effective.
Conclusion
In summary, global variables in MATLAB offer an efficient way to share data across multiple functions. By declaring variables as global, you can simplify your code and improve data management. However, it’s essential to use this feature judiciously, adhering to best practices to maintain code clarity and prevent potential issues. With a solid understanding of how to declare and manage global variables, you can enhance your programming skills and build more efficient MATLAB applications.
FAQ
-
What are global variables in MATLAB?
Global variables are shared variables that can be accessed by multiple functions within the same workspace. -
How do I declare a global variable in MATLAB?
You declare a global variable using theglobal
keyword followed by the variable name in each function that needs access to it. -
What are the advantages of using global variables?
Global variables simplify data sharing between functions, reducing the need for passing variables explicitly. -
Are there any downsides to using global variables?
Yes, overusing global variables can lead to code that is hard to read and debug, and it may introduce naming conflicts. -
What are some best practices for using global variables?
Limit their usage, document your code, avoid naming conflicts, and consider passing variables as function arguments instead.