MATLAB Fprintf Table
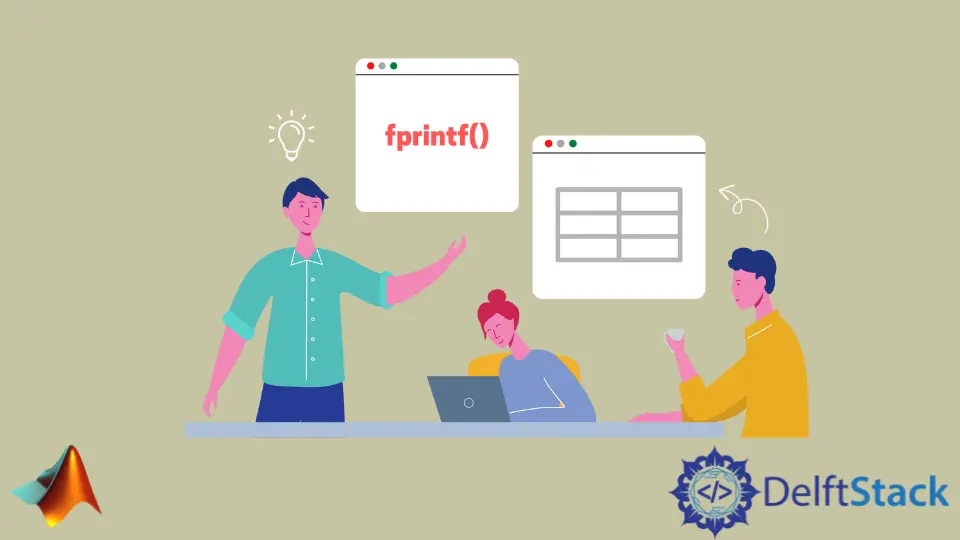
In this tutorial, we will discuss how to print a table using the fprintf()
function in MATLAB.
Print Table Using the fprintf()
Function in MATLAB
The fprintf()
function is used to display formatted text and variables in MATLAB. For example, let’s display some formatted text using this function. See the code below.
age = 22;
fprintf('Sam is %d years old\n',age)
Output:
Sam is 22 years old
In the above code, we are formatting an integer variable. The variable will be printed in place of %d
. You can format any variable type using its conversion character. For example, %d
is used to format an integer, %f
is used to format a float
, etc. Let’s print a table using the fprintf()
function. See the code below.
a = 1.1:0.1:2;
b = 1:10;
data = [b;a];
fprintf('%s\t%s\n','x','y');
fprintf('%d\t%1.1f\n',data);
Output:
x y
1 1.1
2 1.2
3 1.3
4 1.4
5 1.5
6 1.6
7 1.7
8 1.8
9 1.9
10 2.0
In the above code, \t
is used to give a tab space, and \n
is used to move the cursor to a new line. The first conversion character is used to print the first column of the vector, and the second conversion character is used to print the second character. You can print as many variables as you like; just use the appropriate conversion characters for the variables. Check this link for more information about the fprintf()
function.