How to Plot Graph Using the for Loop in MATLAB
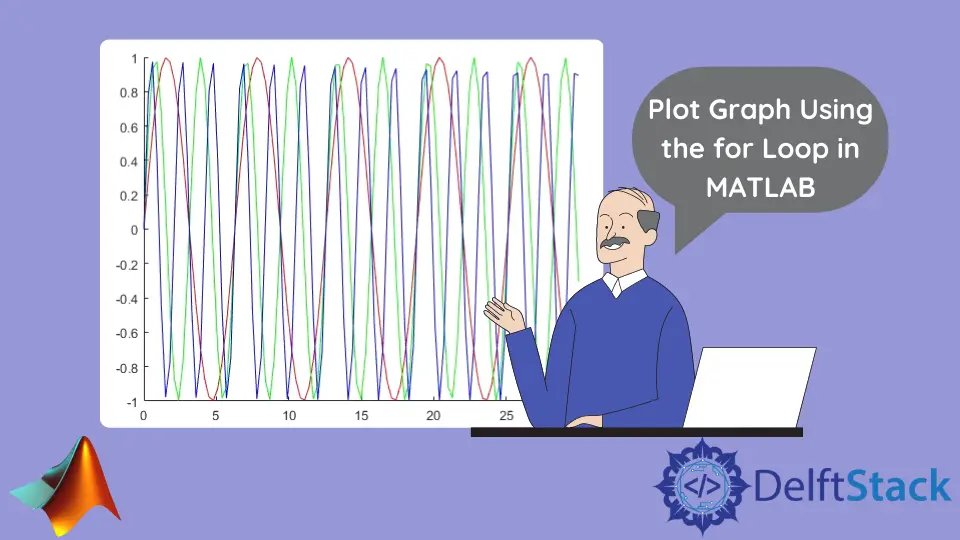
This tutorial demonstrates how to plot a graph using the for
loop in MATLAB.
Plot Graph Using the for
Loop in MATLAB
Usually, the for
loop is not used to plot simple graphs in MATLAB because MATLAB has many functions to plot simple graphs. For example, the doc plot
can be used for plotting graphs, taking several vectors’ matrices as inputs.
Sometimes, the for
loop can plot the graph with several data. For example, if you have several data and want to plot them in a single matrix, you can use the for
loop.
For example, you have two matrices, Ab(L x D)
and Ac(L x D)
, where L
is the length for each data and D
is the amount of different data wanted for the plot.
Let’s try to implement this scenario in MATLAB:
% Create Ab and Ac
Ab=meshgrid(0:0.3:30,1:4);
Ac=zeros(size(Ab));
% Sinusoidals of different frequencies
for k=1:3
Ac(k,:)=sin(k.*Ab(k,:));
end
% colors of the plot
PlotColors = hsv(3);
% Plot
hold on
for k=1:3
plot(Ab(k,:),Ac(k,:),'Color',PlotColors(k,:))
end
hold off
The code above includes two matrices with different data plotted in one matrix using the for
loop. The output plot for the code is:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook