How to Find String in MATLAB
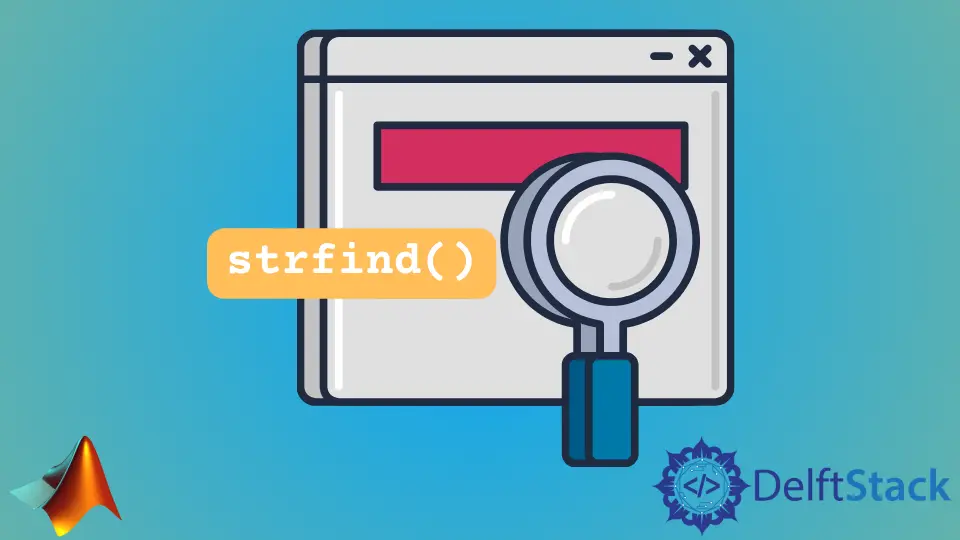
This tutorial will discuss how to find strings within other strings using the strfind()
function in MATLAB.
Find Strings Within Other Strings Using the strfind()
Function in MATLAB
To find occurrences of a string within another string, we can use the strfind()
function in MATLAB. The first argument of the strfind()
function is the string from which you want to find the occurrences of a substring, and the second argument is the string or character you want to find. The output of this function is a vector containing the indices or position of the occurrences of the substring. We can also find the occurrences of a string within an array of strings, and the output will be an array of vectors of indices of the occurrences of the substring. For example, let’s find the occurrences of the space character and the number of spaces within a string using the strfind()
function. See the code below.
v = 'This is a test';
indices_of_spaces = strfind(v,' ')
Number_of_spaces = length(indices_of_spaces)
Output:
indices_of_spaces =
5 8 10
Number_of_spaces =
3
In the above code, we used the length()
function to find the total number of spaces present in the string. In the output, the indices show the position of the space character present in the string. You can change the string and the character you want to find according to your requirements, and if you want to find a specific character or string from an array of strings, you can also find it using this function. Check this link for more details about the strfind()
function.