How to Get Erosion of an Image in MATLAB
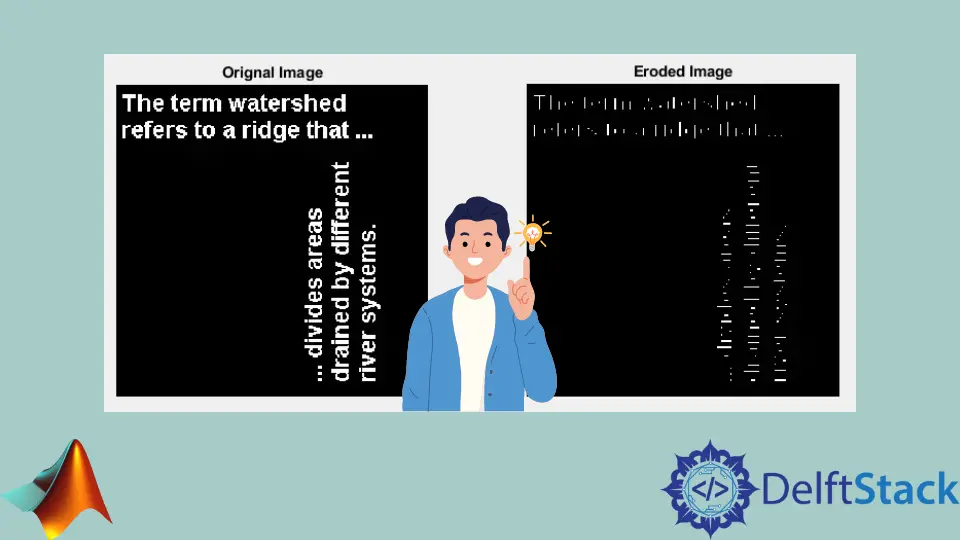
This tutorial will discuss finding the erosion of images using the imerode()
function in Matlab.
Find Erosion of an Image Using the imerode()
Function in MATLAB
Erosion of an image means the shrinking of the image. To find the erosion of an image, we need to move the structuring element over the matrix and replace the pixel’s value with the minimum value of neighbors. Pixel value will be set to zero if the minimum value in the neighborhood is zero. First, we will read the image using the function imread()
and store it in a variable. After that, we need to convert the image values to binary if it’s not already binary by using the function im2bw()
. We also have to create the structuring element. After that, we need to use the imerode()
function, which will check each pixel’s neighbors and replace the pixel value with the minimum value of the pixels. For example, let’s find the erosion of an image using a matrix as a structural element in Matlab and show them in a figure using the subplot()
and inshow()
function. See the code below.
OriginalImg = imread('text.png');
OriginalImg = im2bw(OriginalImg);
ErodMat = ones(3,3);
ErodedImg = imerode(OriginalImg,ErodMat);
figure
subplot(1,2,1)
imshow(OriginalImg)
title('Orignal Image')
subplot(1,2,2)
imshow(ErodedImg)
title('Eroded Image')
Output:
In the above code, we have used a 3-by-3 matrix containing ones to erode the original image. The size of the matrix is directly proportional to the erosion of the image. Increasing the size of the matrix will increase the erosion of the picture. You can use your desired structural element to erode the image. For example, you can use another image to erode an image.