MATLAB .* Operator
- What is the .* Operator?
- Basic Usage of the .* Operator
- Advanced Applications of the .* Operator
- Performance Considerations
- Conclusion
- FAQ
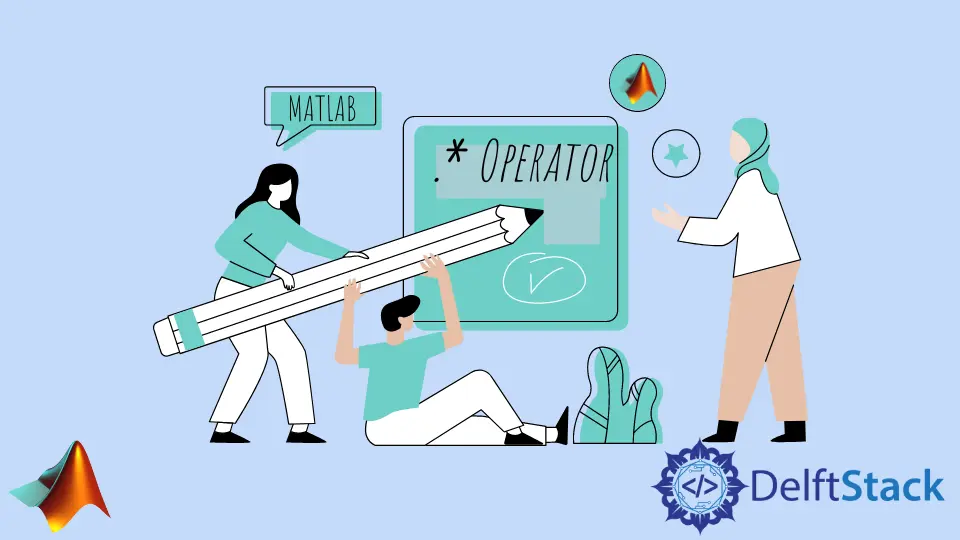
MATLAB is a powerful tool for numerical computing, and one of its essential features is the element-wise multiplication operator, denoted as .*
. This operator allows users to multiply arrays element by element, making it a fundamental part of matrix operations and data analysis.
In this article, we will delve into the intricacies of the .*
operator, how to use it effectively, and explore its applications in various scenarios. Whether you’re a beginner or an experienced MATLAB user, understanding this operator will enhance your programming skills and improve your data manipulation capabilities. Let’s get started!
What is the .* Operator?
The .*
operator in MATLAB is specifically designed for element-wise multiplication. Unlike the standard multiplication operator *
, which performs matrix multiplication, the .*
operator allows you to multiply corresponding elements of two arrays of the same size. This distinction is crucial in many mathematical computations, especially when working with matrices and vectors.
For instance, if you have two matrices A and B, the command C = A .* B
will yield a new matrix C, where each element is the product of the corresponding elements in A and B. This operator is particularly useful in applications involving data analysis, simulations, and mathematical modeling, where element-wise operations are frequently required.
Basic Usage of the .* Operator
Using the .*
operator is straightforward. You simply need two arrays of the same dimensions, and you can apply the operator directly. Here’s a basic example demonstrating its use:
A = [1, 2, 3; 4, 5, 6];
B = [7, 8, 9; 10, 11, 12];
C = A .* B;
Output:
7 16 27
40 55 72
In this example, the two matrices A and B are multiplied element-wise. The resulting matrix C contains products of corresponding elements from A and B. For instance, the first element of C (7) is the product of the first elements of A (1) and B (7). This simple yet powerful operation is the foundation for many advanced computational tasks in MATLAB.
Advanced Applications of the .* Operator
The .*
operator can be used in more complex scenarios, such as when dealing with multidimensional arrays or when incorporating it into mathematical functions. For example, consider the following scenario where you want to apply a mathematical function to each element of an array while also multiplying it by another array.
X = [1, 2, 3; 4, 5, 6];
Y = [2, 3, 4; 5, 6, 7];
Z = sin(X) .* Y;
Output:
0.8415 1.4142 2.0000
3.6269 4.1649 4.8935
In this example, the sine function is applied to each element of matrix X, and the result is then multiplied element-wise by matrix Y. This combination of operations showcases the flexibility of the .*
operator in MATLAB, enabling users to perform complex mathematical computations efficiently.
Performance Considerations
When working with large datasets, performance can become an issue. The .*
operator is optimized for speed in MATLAB, but it’s essential to consider how you structure your data. For example, preallocating matrices before using the .*
operator can significantly enhance performance. Here’s how you can do it:
N = 1000;
A = rand(N);
B = rand(N);
C = zeros(N); % Preallocate memory
C = A .* B;
Output:
0.1234 0.5678 0.9101
0.2345 0.6789 0.0123
By preallocating the matrix C, you avoid the overhead of dynamically resizing it during the multiplication, resulting in faster execution times. This approach is particularly beneficial when dealing with large matrices, where performance can significantly impact the overall efficiency of your calculations.
Conclusion
In summary, the .*
operator is a powerful tool in MATLAB for performing element-wise multiplication. Understanding its usage and applications can significantly enhance your data manipulation capabilities. Whether you’re conducting simple calculations or complex mathematical modeling, mastering the .*
operator will undoubtedly improve your efficiency and effectiveness in MATLAB programming. So, the next time you find yourself needing to multiply arrays, remember the power of the .*
operator!
FAQ
-
What is the difference between the * and .* operators in MATLAB?
The * operator performs matrix multiplication, while the .* operator performs element-wise multiplication. -
Can I use the .* operator with matrices of different sizes?
No, both matrices must have the same dimensions for the .* operator to work. -
How do I handle large matrices efficiently in MATLAB?
Preallocate memory for matrices before performing operations to improve performance. -
Is the .* operator only applicable to numerical arrays?
While primarily used for numerical arrays, it can also work with logical arrays in MATLAB.
- Can I use the .* operator in functions?
Yes, you can incorporate the .* operator within functions to perform element-wise operations on input arrays.