How to Display String in MATLAB
-
Displaying a String Using the
disp()
Function in MATLAB -
Display a String Using
disp()
Function in MATLAB
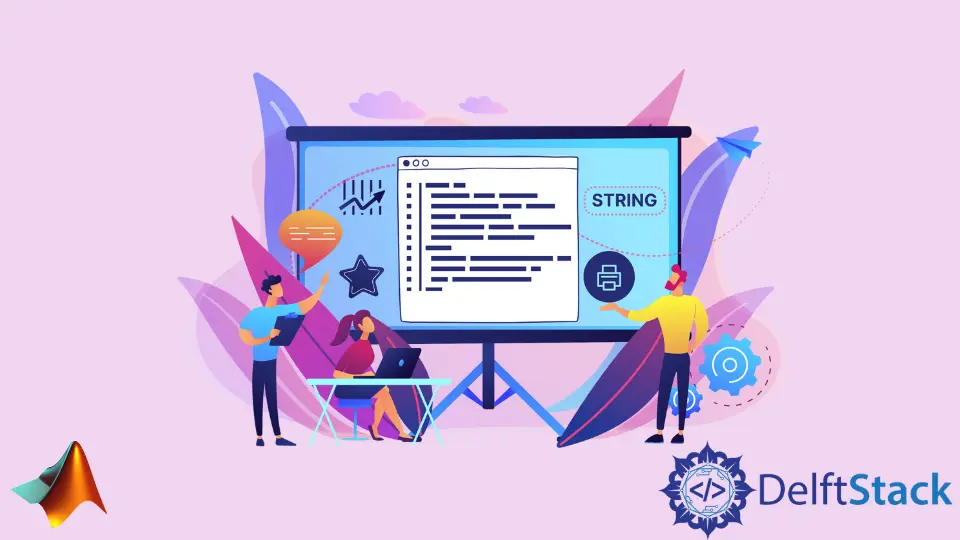
This tutorial will introduce how to use the disp()
and sprintf()
function to display a string in MATLAB.
Displaying a String Using the disp()
Function in MATLAB
You can use the disp()
function to display a string in MATLAB. For example, let’s display a variable containing a string. See the below code.
str = "Hello World";
disp(str)
Output:
Hello World
In the above code, we display a variable str
which contains a string. We can also join multiple strings in an array and display them using the disp()
function. See the below code.
name = 'Sam';
age = 25;
str = [name, ' is ', num2str(age), ' years old.'];
disp(str)
Output:
Sam is 25 years old.
Make sure to change every variable to char
to avoid errors.
Display a String Using disp()
Function in MATLAB
You can use the sprintf()
function to display a string in MATLAB. For example, let’s display a variable containing a string in MATLAB. See the below code.
str = "Hello World";
sprintf("%s",str)
Output:
ans =
"Hello World"
We can format the variable and then display it using the sprintf()
function just like the fprintf()
in the C
language. For example, let’s format a floating-point number using the sprintf()
function. See the below code.
f = 1.1234;
sprintf("%0.2f",f)
Output:
ans =
"1.12"
In the above code, we display a floating-point number up to two decimal places.