MATLAB Data Types
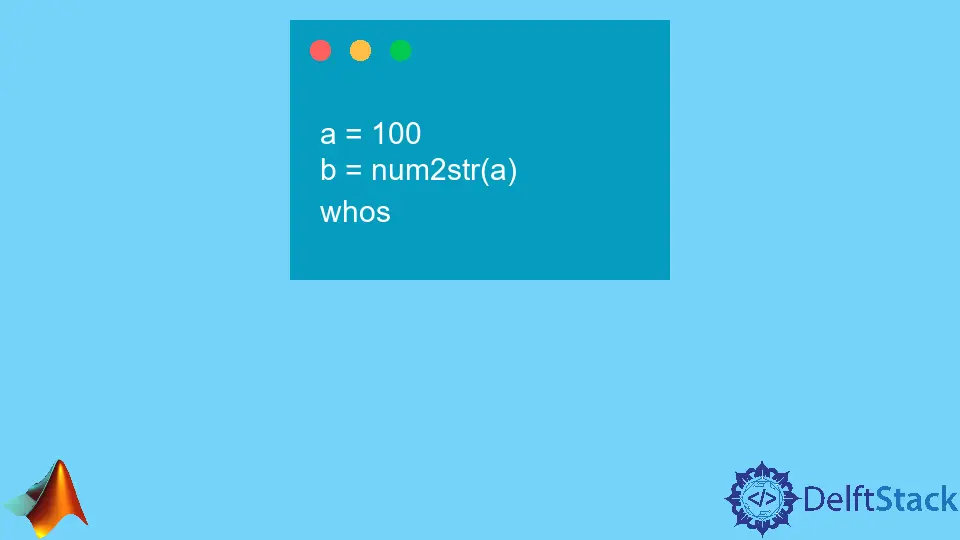
This tutorial will discuss storing different data types in a variable in Matlab.
MATLAB Data Types
Data can be stored in different types like character, string, double, integer, cell array, etc.
In Matlab, we don’t have to define the data type. We have to pass the value inside a variable, and Matlab will allocate it some memory. We have to use symbols when we store the data in a variable.
For example, if we want to store a string in a variable, we must use double quotation marks.
If we need to store a character in a variable, we must use single quotation marks.
If we need to store numbers, we don’t have to use any symbols.
To store cell array data, we have to use curly brackets. To store a vector or matrix, we have to use square brackets.
We can use the whos
command to check the data type, class, or variables stored in the workspace.
Let’s store different data types inside some variables. See the code below.
a = 100;
b = 'char';
c = "String2";
e = [1 2 3];
f = {'a',2,"s"};
whos
Output:
Name Size Bytes Class Attributes
a 1x1 8 double
b 1x4 8 char
c 1x1 150 string
e 1x3 24 double
f 1x3 472 cell
The output shows that the variables have different data types or classes.
Matlab also has other data types like categorical arrays, tables, structures, and time series.
Categorical arrays contain qualitative data with values from a set of discrete data.
Tables contain arrays in tabular form in which different columns have different names and values.
Structures include arrays with named fields that contain data of varying types and sizes.
We can convert one data type or class to another data type or class using Matlab’s built-in functions.
For example, we can use the num2str()
to convert numbers to a character array, the int2str()
function to convert integer to string or character array, the str2num()
function to convert string or character array to numeric array, and the str2double()
function to convert string or character array to double data type.
Let’s convert a number to a character using the num2str()
function. See the code below.
a = 100
b = num2str(a)
whos
Output:
a =
100
b =
'100'
Name Size Bytes Class Attributes
a 1x1 8 double
b 1x3 6 char
Variable a is of class double in the output, but the number is now of class char after conversion.