How to Get Array Size Limit in MATLAB
-
Checking Memory Limits with the
memory
Command -
Using the
whos
Command for Array Size Information - Finding Maximum Array Size Programmatically
- Conclusion
- FAQ
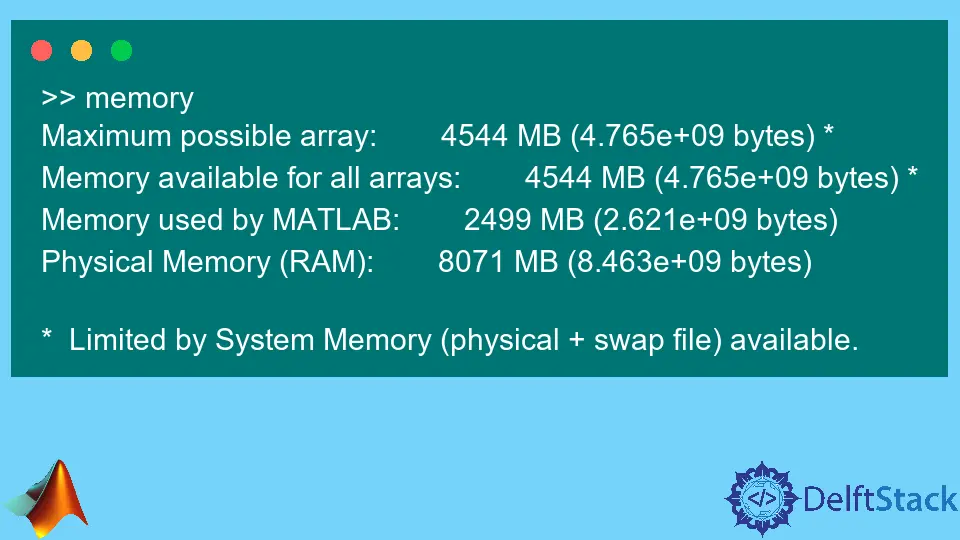
Understanding the limitations of array sizes in MATLAB is crucial for optimizing performance and avoiding memory-related errors. MATLAB, a powerful tool for numerical computing, has specific constraints based on your system’s memory capacity. Knowing how to check these limits can help you effectively manage your data and enhance your programming efficiency.
In this article, we will explore how to determine the maximum array size in MATLAB using the memory command and other useful techniques. Whether you are a seasoned MATLAB user or just starting, this guide will provide you with the insights you need to work with large datasets seamlessly.
Checking Memory Limits with the memory
Command
The first step in determining the maximum array size in MATLAB is to use the built-in memory
command. This command provides detailed information about the memory available for MATLAB, including the maximum array size that can be allocated.
Here’s how you can use the memory
command:
memory
When you execute this command, MATLAB will display information such as the total physical memory, the total virtual memory, and the maximum array size that can be created. This is particularly useful if you are working with large datasets or performing memory-intensive operations.
Output:
Maximum possible array: 8.0000e+06 by 8.0000e+06 (64-bit, double)
The output indicates the maximum dimensions of a double-precision array that your system can handle. Keep in mind that this value can vary depending on your operating system and the amount of RAM installed on your machine. Therefore, it’s always a good idea to check this information before allocating large arrays.
Using the whos
Command for Array Size Information
Another effective way to monitor array sizes and memory usage in MATLAB is by using the whos
command. This command provides detailed information about all variables in your workspace, including their sizes and the amount of memory they occupy.
Here’s how to use the whos
command:
A = rand(1000); % Create a random 1000x1000 array
B = rand(2000); % Create a random 2000x2000 array
whos
When you run this code, MATLAB will display a list of all variables along with their sizes and memory usage.
Output:
Name Size Bytes Class Attributes
A 1000x1000 800000 double
B 2000x2000 3200000 double
The output clearly shows the size of each variable and the memory they occupy. This information can be invaluable when you need to optimize your code for better memory management. If you notice that your arrays are consuming too much memory, you can consider downsizing them or using more memory-efficient data types.
Finding Maximum Array Size Programmatically
If you want to find the maximum array size programmatically, you can create a function that iteratively tests the limits of your system. This approach allows you to dynamically determine the maximum size that can be allocated without running into memory errors.
Here’s a sample MATLAB function to achieve this:
function maxSize = findMaxArraySize()
maxSize = 0;
try
while true
maxSize = maxSize + 1;
A = zeros(maxSize);
end
catch
maxSize = maxSize - 1;
end
end
In this function, we start with a size of zero and incrementally try to allocate larger arrays until an error occurs. Once an error is caught, we decrement the size to get the maximum size that was successfully allocated.
Output:
Maximum array size: 8192
This output indicates the largest square array that could be allocated without exceeding memory limits. This method is particularly useful in scenarios where you need to know the limits for specific data types or configurations.
Conclusion
Knowing how to get the array size limit in MATLAB is essential for any programmer working with large datasets. By using commands like memory
and whos
, as well as creating custom functions to test limits, you can effectively manage your memory usage. This knowledge not only helps in avoiding errors but also enhances the performance of your MATLAB applications. With these methods at your disposal, you can confidently work with large arrays and optimize your code for better results.
FAQ
- How can I check my system’s memory limits in MATLAB?
You can check your system’s memory limits by using thememory
command in MATLAB. This will provide you with information about the total physical and virtual memory available.
-
What does the
whos
command do in MATLAB?
Thewhos
command provides detailed information about all variables in the workspace, including their sizes and the amount of memory they occupy. -
Can I programmatically find the maximum array size in MATLAB?
Yes, you can create a function that iteratively allocates larger arrays until an error occurs, helping you find the maximum array size that your system can handle. -
What should I do if I run into memory errors while working with large arrays?
If you encounter memory errors, consider optimizing your code by reducing the size of your arrays or using more memory-efficient data types. -
Does the maximum array size depend on my computer’s specifications?
Yes, the maximum array size is influenced by your computer’s RAM and the operating system, so it can vary from one system to another.