How to Create Animated Plot in MATLAB
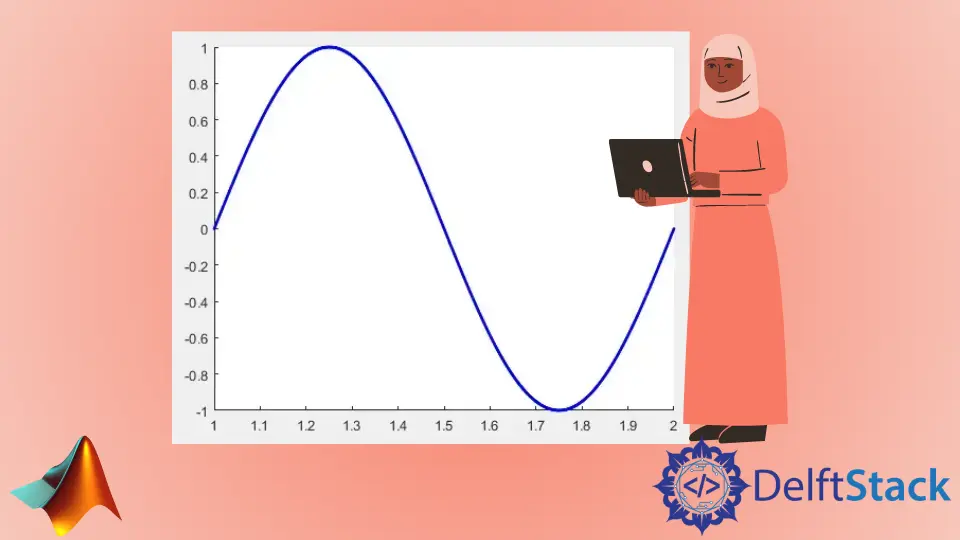
This tutorial will introduce how to draw an animated plot using the drawnow
command and pause()
function in MATLAB.
Draw an Animated Plot Using the drawnow
Command and pause()
Function in MATLAB
If you want to make an animated plot and see the plot being made in real-time, you can use a loop and drawnow
command. The drawnow
command updates figures on each callback. To draw an animated plot, you have to use it inside a loop to plot one variable in one iteration and update the figure using the drawnow
command. For example, let’s draw the animated plot of a sine wave. See the code below.
t = 1:0.001:2;
x = sin(2*pi*t);
figure
hold on
axis([1 2 -1 1])
for i=1:numel(t)
plot(t(i),x(i),'.','Color','b')
drawnow
end
Output:
You can choose different options depending on your requirements. You can change the axis limits using the axis
function. You can change the plot color using the Color
property and plot marker. If you want to change the timing of the animation, you can use the pause()
function instead of the drawnow
command to give the animation your desired animation time. You can pass the time in seconds inside the pause()
function. So a best practice will be to use a value in milliseconds; otherwise, the animation will be very slow.