How to Make a Column Vector in MATLAB
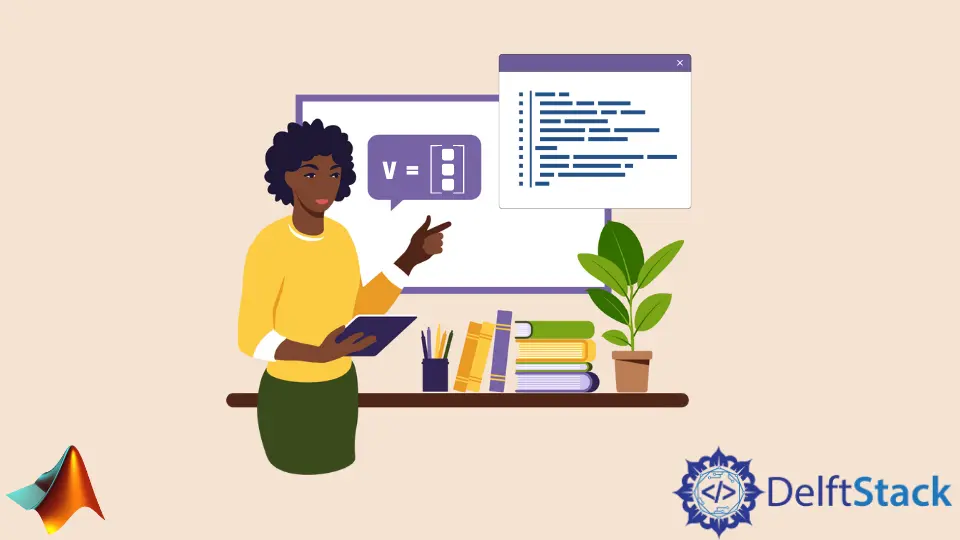
This tutorial will discuss creating a column vector using the semicolon symbol in MATLAB.
Creating Column Vectors Using the Semicolon Symbol in MATLAB
A column vector is a vector that has only one column. To create a column vector in MATLAB, we must use the semicolon symbol after each element except the last element. For example, let’s create a column vector with three elements using the semicolon symbol. See the code below.
v = [1;2;3]
Output:
v =
1
2
3
We can also take the transpose of a row vector to convert it into a column vector. For example, let’s create a row vector and convert it into a column vector using the transpose()
function. See the code below.
RowV = [1 2 3]
colV = transpose(RowV)
Output:
RowV =
1 2 3
colV =
1
2
3
We can also use the apostrophe symbol instead of the transpose()
function to take the transpose of a vector or matrix. For example, we can change the third line of the above code to RowV
, generating the same result. We can also convert a matrix into a column vector using the colon symbol. For example, let’s create a matrix and convert it into a column vector using the colon symbol. See the code below.
RowV = [1 2 3; 7 8 9]
colV = RowV(:)
Output:
RowV =
1 2 3
7 8 9
colV =
1
7
2
8
3
9
In the output, the columns of the given matrix are placed vertically on top of each other to create a single column vector. The first column will be on top, and the second column will be below the first column, and so on.