How to Extract Frames From Video Using MATLAB
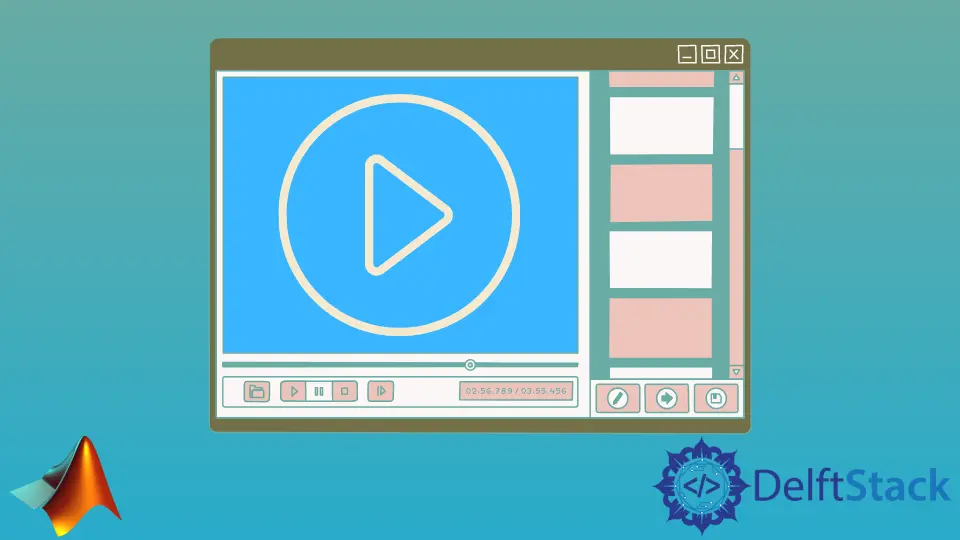
This tutorial will discuss extracting the frames of a video using read()
function in Matlab.
Extract Frames From a Video Using read()
Function in MATLAB
A video is composed of many frames. In other words, it is composed of pictures, so when images or frames get repeated one after the other, we will see a video playing. For example, suppose a video is composed of 60 frames per second, which means there will be 60 frames in a single second. The frames change so fast that we only see a video playing. First of all, we need to read the video in Matlab using the function VideoReader()
. After that, we need to find the total number of frames present in the video using the NumberOfFrames
property. We will use a for loop, which will start from 1 and end at the max number of frames in the video. Now we will find each frame present in the video and show them on a plot using the function subplot()
and imshow()
. For example, let’s read a video and extract all of its frames in Matlab. See the code below.
v = VideoReader('bon_fire_dog_2.mp4');
totalFrames = v.NumberOfFrames;
NFP = ceil(sqrt(totalFrames));
for i=1:totalFrames
frame = read(v,i);
subplot(NFP,NFP,i)
imshow(frame)
end
Output:
In the above code, the variable NPF
sets the number of rows and columns in the above figure. We can also save each frame as an image inside a folder using the imwrite()
function. See the code below.
v = VideoReader('bon_fire_dog_2.mp4');
totalFrames = v.NumberOfFrames;
NFP = ceil(sqrt(totalFrames));
for i=1:totalFrames
frame = read(v,i);
ImgName = strcat(int2str(i),'.png');
imwrite(frame, ImgName);
end
The above code will save all the frames present in the video as images with .png
file format inside the same directory as the script file. You can also change the file name and the file extension of the image. If you don’t want to extract all the frames from the video, and you want to extract only the first frame of the video. To do that, you have to change the second argument of the read()
function to 1 like read(v,1)
. If you want to extract the last frame of the video, you have to change the second argument to inf
like read(v,inf)
. If you want to extract a specific range of frames from the video, you have to change the second argument to vector. For example, you can use the read(v,[5 10])
function to read the frames from 5 to 10 from the video v
.